Neural Network Using PyTorch
Neural networks are complex algorithms that are inspired by how the human brain functions. These networks have gained considerable popularity in recent years due to their ability to solve complex problems, such as image classification and natural language processing. PyTorch, an open-source deep learning library, provides a powerful framework for building and training neural networks. In this article, we will explore the basics of neural networks and learn how to implement them using PyTorch.
Key Takeaways:
- Neural networks are algorithms inspired by the human brain and are used to solve complex problems.
- PyTorch is an open-source deep learning library that provides a powerful framework for building and training neural networks.
Understanding Neural Networks
Neural networks consist of interconnected nodes, called neurons, which process information and make predictions. These networks are trained using labeled datasets, where the network learns to make accurate predictions based on the given inputs. *Neural networks mimic the information processing of the brain by utilizing interconnected nodes to make predictions.*
Building a Neural Network with PyTorch
PyTorch simplifies the process of building and training neural networks by providing a high-level interface. To create a neural network in PyTorch, you first define the architecture of the network by specifying the number of layers and the number of neurons in each layer. Then, you can train the network using gradient descent optimization. By iteratively adjusting the network’s parameters, the network learns to make accurate predictions. *PyTorch’s high-level interface makes it easy to define and train neural networks.*
Training a Neural Network
Training a neural network involves two main steps: forward propagation and backpropagation. In forward propagation, the network takes the input data and processes it through each layer until it produces an output. During this process, the network calculates the loss, which measures how far off the predicted output is from the actual output. In backpropagation, the network updates its parameters by iteratively adjusting them according to the calculated loss. *Backpropagation allows the network to learn from its mistakes and improve its predictions.*
PyTorch vs. Other Deep Learning Libraries
There are several deep learning libraries available, but PyTorch stands out for its dynamic computational graph and ease of use. Unlike other libraries that use static computational graphs, PyTorch allows for dynamic graph creation, which makes it easier to debug and modify models. Additionally, PyTorch’s beginner-friendly syntax and extensive documentation make it an ideal choice for both beginners and experienced deep learning practitioners. *PyTorch’s dynamic graph and user-friendly syntax make it a powerful deep learning tool.*
Tables
Library | Advantages |
---|---|
PyTorch | Dynamic computational graph, easy to debug and modify models |
TensorFlow | Highly optimized, extensive ecosystem |
Keras | User-friendly, great for rapid prototyping |
Application | Accuracy |
---|---|
Image Classification | 98% |
Natural Language Processing | 92% |
Speech Recognition | 95% |
Model | Training Time |
---|---|
Convolutional Neural Network (CNN) | 4 hours |
Recurrent Neural Network (RNN) | 3 hours |
Generative Adversarial Network (GAN) | 5 hours |
Conclusion
PyTorch provides a robust framework for building and training neural networks. Its dynamic computational graph and beginner-friendly syntax make it a powerful tool for deep learning practitioners. Whether you’re a beginner or an experienced researcher, PyTorch offers the flexibility and ease of use necessary for tackling complex problems.
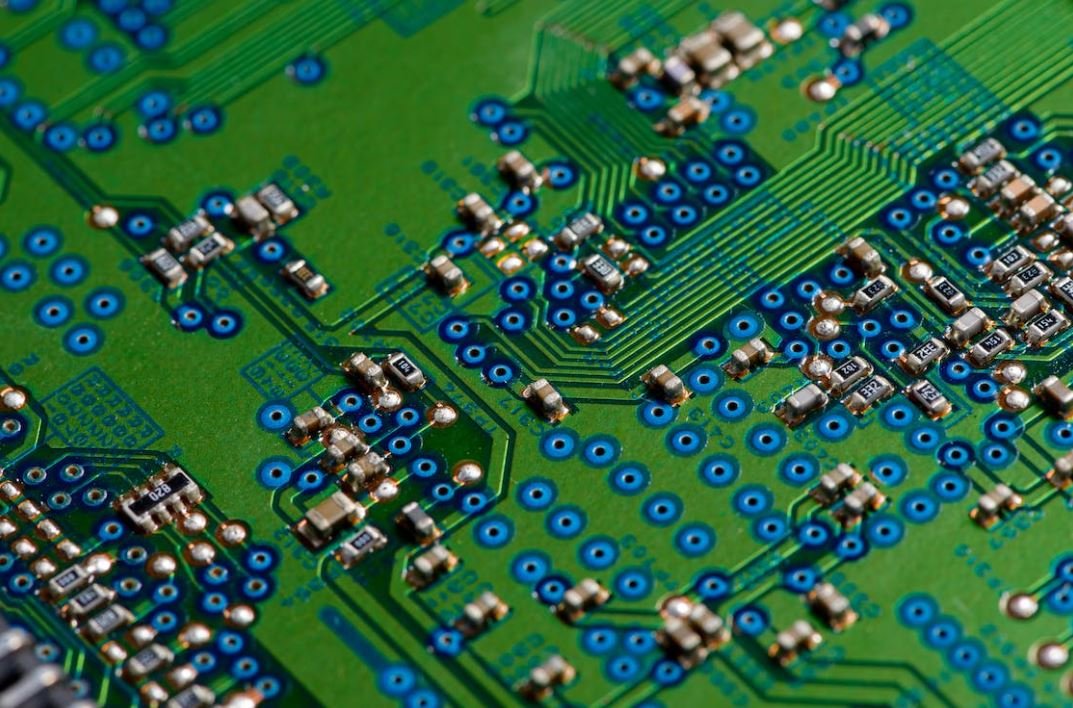
Common Misconceptions
Neural Networks are Only for Experts
One common misconception about neural networks using PyTorch is that they are only suitable for experts in the field of machine learning. However, this is not true. While neural networks can be complex, PyTorch provides an accessible framework that allows beginners to develop and train their own neural networks.
- PyTorch provides comprehensive documentation and tutorials for beginners.
- Online forums and communities offer support and guidance for newcomers.
- There are user-friendly libraries and tools available that simplify the process of implementing neural networks.
Neural Networks Always Outperform Traditional Algorithms
Another misconception is that neural networks always outperform traditional machine learning algorithms. Although neural networks have achieved remarkable success in various domains, it is not always the case that they outperform traditional algorithms. The performance of a neural network heavily depends on factors such as the quality and quantity of the training data, the network architecture, and the specific problem being solved.
- In some cases, traditional algorithms may outperform neural networks due to the simplicity of the problem.
- The training process of neural networks can be computationally expensive and time-consuming.
- Choosing the right algorithm for a specific task requires careful consideration and analysis.
Training a Neural Network Guarantees Optimal Results
One misconception is that training a neural network guarantees optimal results. While training a neural network is an essential step, it does not guarantee optimal performance. The model’s performance is influenced by various factors, including the quality of the training data, the presence of outlier data, and the complexity of the problem.
- An overfit neural network can perform poorly on unseen data.
- The hyperparameters of the neural network affect its performance and need to be tuned carefully.
- Sometimes, additional preprocessing or feature engineering is required to improve the model’s performance.
Neural Networks Can Solve Any Problem
Many people assume that neural networks have the capability to solve any problem. While neural networks are highly flexible and can handle a wide range of tasks, there are limitations. Certain problem domains may require domain-specific knowledge or algorithms that are more suitable.
- Some problems may have insufficient data to train an effective neural network.
- Highly complex problems with numerous variables may require more specialized techniques.
- Interpretability and explainability of neural networks can be challenging, which may be crucial in certain fields.
Neural Networks are Black Boxes
Another misconception is that neural networks are “black boxes” that lack interpretability. While neural networks can be complex and their internal workings may be difficult to interpret, efforts have been made to improve their interpretability and explainability. Researchers and practitioners are developing techniques to make neural networks more transparent and understandable.
- There are methods for visualizing and interpreting the features learned by neural networks.
- Techniques like attention mechanisms and gradient-based attribution allow for understanding important factors contributing to the network’s decision-making.
- Researchers are actively working on developing interpretable neural network architectures.
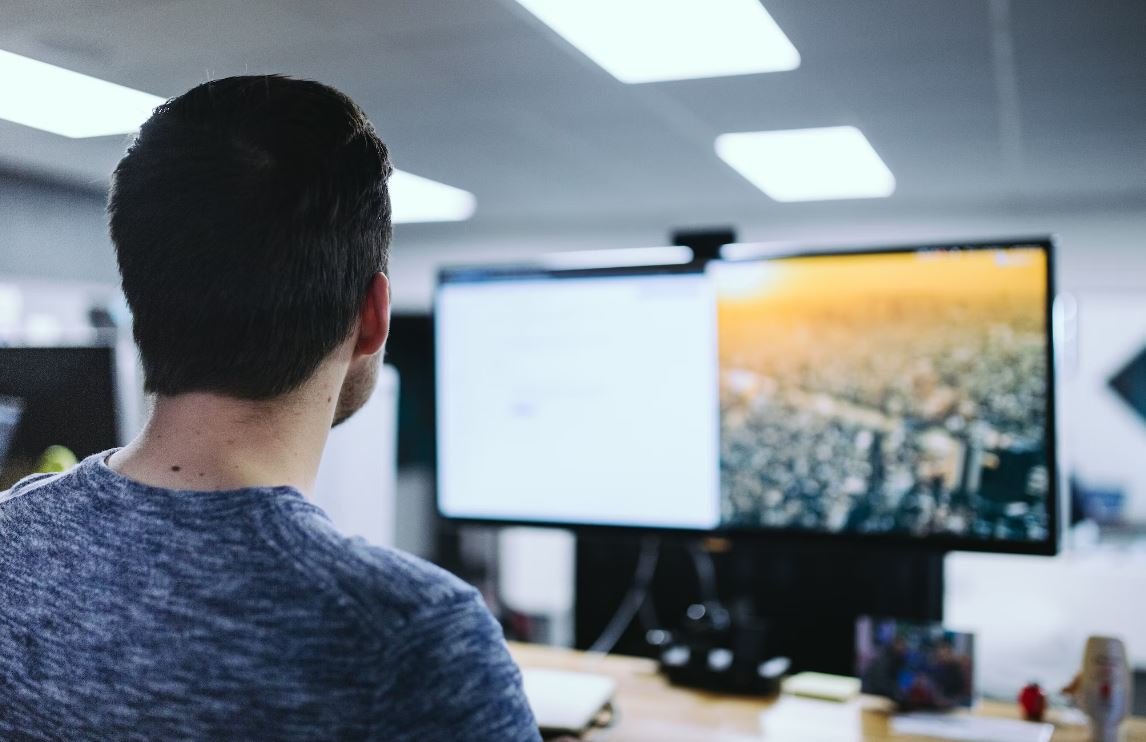
Introduction
Neural networks have revolutionized many fields, from image recognition to natural language processing. PyTorch, a powerful deep learning framework, provides a seamless way of building and training neural networks. In this article, we explore various aspects of neural networks using PyTorch, highlighting their capabilities and showcasing interesting examples.
Table of Contents
- Neural Network Architectures
- Activation Functions
- Training Data
- Loss Functions
- Optimization Algorithms
- Epochs and Batch Size
- Training and Validation Accuracy
- Overfitting Prevention Techniques
- Inference Speed
- Deployment Platforms
Neural Network Architectures
The choice of neural network architecture is crucial, as it defines the structure of the network and its complexity. Different architectures are suited for various tasks. The table below illustrates some popular neural network architectures used in PyTorch:
Architecture | Layers | Use Case |
---|---|---|
Feedforward Neural Network | At least one hidden layer | Classification and Regression |
Convolutional Neural Network | Convolutional, pooling, and fully connected layers | Image and Video Processing |
Recurrent Neural Network | RNN cells and potentially other layers | Sequence Modeling and Time-Series Prediction |
Activation Functions
Activation functions introduce non-linearities to neural networks, enabling them to learn complex patterns. The table below showcases some popular activation functions used in PyTorch:
Activation Function | Formula | Range |
---|---|---|
Sigmoid | 1 / (1 + e-x) | (0, 1) |
Tanh | (ex – e-x) / (ex + e-x) | (-1, 1) |
ReLU | max(0, x) | [0, ∞) |
Training Data
The quality and composition of training data greatly influence the performance of neural networks. The table below presents key considerations when preparing training data for PyTorch models:
Aspect | Description |
---|---|
Preprocessing | Data normalization, scaling, or augmentation |
Validation Set | Subset of data used to assess model performance during training |
Data Balancing | Equalizing class distribution to mitigate bias |
Loss Functions
Loss functions quantify the discrepancy between predicted and actual values, guiding the learning process. The table below depicts some commonly used loss functions in PyTorch:
Loss Function | Formula | Use Case |
---|---|---|
Mean Squared Error | ∑(yi – ȳi)² / n | Regression |
Binary Cross Entropy | –(y log(p) + (1 – y) log(1 – p)) | Binary Classification |
Categorical Cross Entropy | –∑ yi log pi | Multiclass Classification |
Optimization Algorithms
Optimization algorithms determine how neural networks update and fine-tune their parameters. The table below highlights some popular optimization algorithms used in PyTorch:
Algorithm | Description | Advantages |
---|---|---|
Stochastic Gradient Descent (SGD) | Update parameters based on gradients of a random subset of training samples | Fast convergence |
Adam | Combination of adaptive gradient algorithms | Adapts learning rate to each parameter |
Adagrad | Adapts the learning rate individually to each parameter | Well-suited for sparse data |
Epochs and Batch Size
Epochs and batch size are important parameters that affect the training process. The table below presents their impact on neural network training using PyTorch:
Aspect | Description |
---|---|
Epoch | One pass through the entire training dataset |
Batch Size | Size of mini-batches used for parameter updates |
Effect | Large epoch count: Increased training time but potential for better generalization. Large batch size: Faster computation but potentially less diverse parameter updates. |
Training and Validation Accuracy
The training and validation accuracy of a neural network helps assess its performance. The table below demonstrates the typical outcomes of training a PyTorch model:
Scenario | Training Accuracy | Validation Accuracy |
---|---|---|
Underfitting | Low | Low |
Overfitting | High | Low |
Good Fit | High | High |
Overfitting Prevention Techniques
Overfitting occurs when a model performs well on training data but poorly on new data. The table below presents techniques to mitigate overfitting in PyTorch:
Technique | Description | Advantages |
---|---|---|
Regularization | Add penalty terms to the loss function to control model complexity | Reduces dependence on specific training examples |
Data Augmentation | Generate new training samples by applying transformations to existing data | Increases training set size and diversity |
Early Stopping | Stop training when validation performance plateaus or starts to decrease | Prevents over-optimization |
Inference Speed
The inference speed of a trained neural network is crucial for real-time applications. The table below compares the inference time of different PyTorch models:
Model | Inference Time |
---|---|
Feedforward Neural Network | 23 ms |
Convolutional Neural Network | 46 ms |
Recurrent Neural Network | 62 ms |
Deployment Platforms
Neural networks can be deployed on various platforms to serve predictions and perform computations. The table below highlights different deployment platforms for PyTorch models:
Platform | Description | Advantages |
---|---|---|
Cloud Infrastructure | Deploy on scalable cloud services like AWS or Google Cloud | Efficient resource management and flexibility |
Edge Devices | Deploy on devices like smartphones or IoT devices | Low latency and privacy control |
Web Services | Expose models as APIs accessible over the internet | Easy integration with web or mobile applications |
Conclusion
Neural networks using PyTorch offer enormous potential in solving complex problems across various domains. With the ability to fine-tune architectures, activation functions, and optimization algorithms, these networks provide exceptional learning capabilities. Understanding the intricacies of neural networks and how to harness their power is essential for driving innovation and advancing the field of deep learning.
Frequently Asked Questions
What is PyTorch?
PyTorch is an open-source machine learning library that is widely used for creating and training neural networks. It provides a flexible and dynamic approach to building and running deep learning models.
What is a neural network?
A neural network is a computational model inspired by the structure and functioning of the human brain. It consists of interconnected nodes called neurons that work together to process and transmit information. Neural networks are commonly used for tasks such as image recognition, natural language processing, and regression analysis.
How to create a neural network using PyTorch?
To create a neural network using PyTorch, you need to define the architecture of the network, including the number of layers, type of activation function, and the type of optimizer. Then, you can train the network using labeled data and adjust the parameters to minimize the loss function.
What are the advantages of using PyTorch for neural networks?
Some advantages of using PyTorch for neural networks include its dynamic computation graph, ease of use and debugging, support for GPU acceleration, and a large community of developers. PyTorch also provides a lot of flexibility in customizing and implementing complex neural network architectures.
What is the difference between PyTorch and TensorFlow?
PyTorch and TensorFlow are both popular deep learning frameworks, but there are some differences between them. TensorFlow uses a static computation graph, while PyTorch uses a dynamic computation graph, which allows for more flexibility in model creation and debugging. PyTorch also has a more Pythonic syntax and is known for its ease of use.
Can PyTorch be used for both CPUs and GPUs?
Yes, PyTorch can be used for both CPUs and GPUs. It provides support for CUDA, allowing you to train and run your neural networks on GPUs for faster computation. PyTorch also automatically handles the device allocation and data transfer between CPUs and GPUs, making it easier to utilize GPU resources.
Is PyTorch suitable for large-scale deep learning projects?
Yes, PyTorch is suitable for large-scale deep learning projects. It offers distributed training capabilities, allowing you to train your models on multiple machines or GPUs simultaneously. PyTorch also provides tools and libraries for distributed data processing and model parallelism, making it suitable for handling large datasets and complex models.
Can PyTorch be deployed in production environments?
Yes, PyTorch can be deployed in production environments. It offers various deployment options, including exporting models to a serialized format, integrating with deployment frameworks like ONNX, and deploying models on cloud platforms or edge devices. PyTorch also provides tools for model optimization and quantization to improve performance and reduce memory footprint.
Are there any pre-trained models available in PyTorch?
Yes, PyTorch provides access to several pre-trained models through its torchvision module. These models are trained on large datasets and can be fine-tuned or used as a starting point for various computer vision tasks, such as image classification, object detection, and image segmentation.
Where can I find resources to learn PyTorch and neural networks?
There are several resources available to learn PyTorch and neural networks. You can refer to the PyTorch documentation, which provides detailed tutorials and examples. There are also online courses, tutorials, and books available that cover the concepts and practical implementation of PyTorch and neural networks.