Input Data Validation in Spring Boot
In Spring Boot, input data validation is an essential part of building robust and secure applications. Validating user input helps prevent potential security vulnerabilities and ensures data integrity within your system. This article will explore various data validation techniques in Spring Boot and how to implement them effectively.
Key Takeaways
- Input data validation is crucial for building secure applications.
- Spring Boot provides built-in support for data validation.
- Using proper validation techniques leads to improved data integrity.
Introduction
Data validation is the process of ensuring that the input provided by users adheres to predefined rules and constraints. By validating the data, we can ensure its correctness, completeness, and suitability for further processing. In Spring Boot, data validation can be easily implemented using the validation features provided by the Spring Framework.
With input data validation, we can prevent potential security vulnerabilities and improve data quality within our applications.
Validation Annotations
Spring Boot offers several validation annotations that can be applied to input fields to enforce validation rules. These annotations are part of the Java Bean Validation standard.
- @NotNull: Validates that the annotated field is not null.
- @NotBlank: Validates that the annotated string is not null or empty.
- @Size(min, max): Validates that the annotated field’s size is within the specified limits.
- @Pattern(regexp): Validates that the annotated string matches the specified regular expression.
Using these annotations, we can easily define rules for validating input data within our Spring Boot applications.
Custom Validators
In addition to the built-in validation annotations, Spring Boot allows us to create our own custom validators to handle more complex validation scenarios. To create a custom validator, we need to implement the Validator interface and override its validate method.
Creating custom validators gives us greater flexibility in defining and enforcing validation rules specific to our application’s requirements.
Validation Error Handling
When the input data fails validation, Spring Boot provides mechanisms for handling validation errors. By default, validation errors are reported as BindingResult objects. These objects contain information about the validation errors and the validated object.
Proper handling of validation errors ensures that users receive meaningful feedback regarding their input, improving the overall user experience.
Validation Example
Let’s consider an example where we have a registration form with multiple fields, including a date of birth field. We want to ensure that the date of birth entered by the user is in the past.
We can define a custom validator for our registration form, which implements the Validator interface. Within the validator, we can use the @Past annotation to validate the date of birth field.
Here is an example of how the validation might be implemented:
Field | Validation Annotation |
---|---|
Date of Birth | @Past |
Conclusion
Data validation plays a critical role in ensuring the integrity and security of our applications. In Spring Boot, we have access to a range of validation techniques, including built-in annotations and custom validators. By implementing these techniques effectively, we can improve the overall reliability and trustworthiness of our applications.
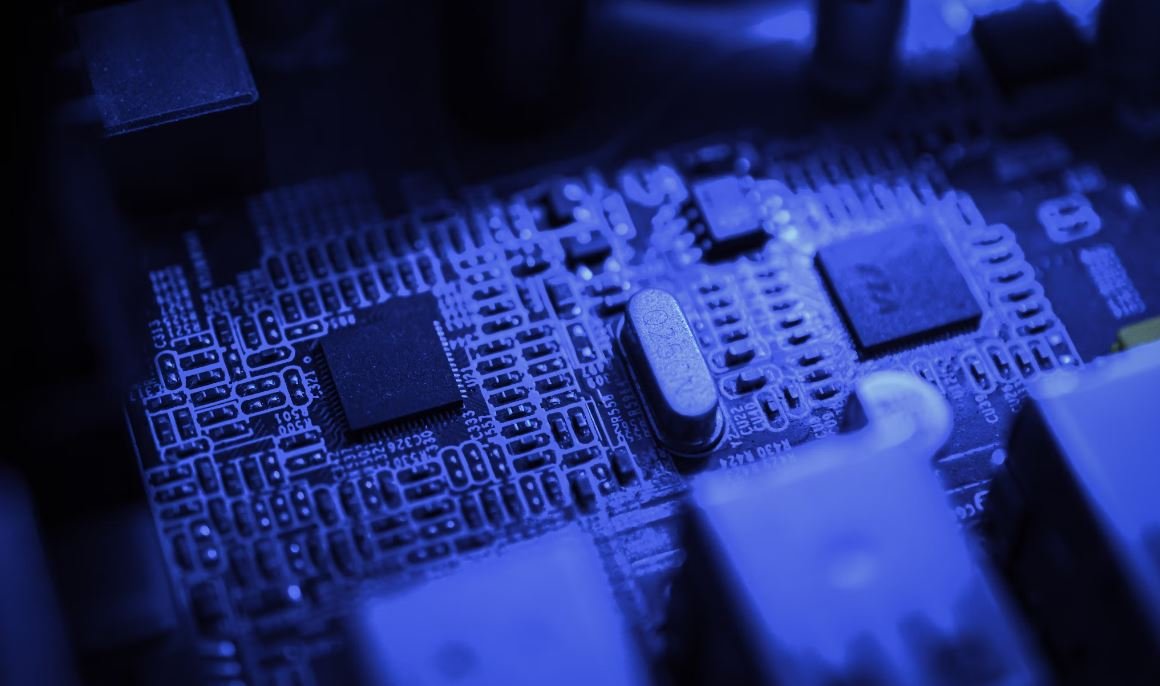
Common Misconceptions
Misconception 1: Input Data Validation is not necessary with Spring Boot
One common misconception people have is that input data validation is not necessary when using Spring Boot. However, this is far from the truth. While Spring Boot does provide some built-in validation mechanisms, it is still important to implement additional input data validation to ensure the integrity and security of your application.
- Spring Boot’s built-in validation may not cover all possible cases
- Input data validation can help prevent security vulnerabilities
- Additional validation can ensure data consistency and accuracy
Misconception 2: Input Data Validation slows down the application
Another misconception is that implementing input data validation in Spring Boot can significantly slow down the application. While it is true that validation does add some overhead, when done properly, the impact on performance is minimal and the benefits far outweigh the costs.
- Proper implementation and caching techniques can minimize performance impact
- Validation is crucial for maintaining data integrity and preventing errors
- The cost of potential security breaches far exceeds the performance impact of validation
Misconception 3: Framework-level validation is sufficient
Some people believe that relying solely on framework-level validation, such as using annotations like @NotNull or @Valid, is enough to ensure input data validation. However, this is a misconception as it may not cover all validation cases specific to your application.
- Framework-level validation may not cover custom validation requirements
- Additional validation logic may be needed depending on the business rules
- Custom validation can provide more detailed error messages to users
Misconception 4: Input data validation is a one-time process
One common misconception is that input data validation is only performed once, usually at the point of user input. However, it is important to note that input data can change over time and may need to be revalidated at various stages in the application.
- Input data validation should be performed at multiple stages in the application flow
- Data may become invalid due to changes in business rules or system updates
- Real-time validation can provide a better user experience by catching errors early
Misconception 5: Input data validation is only needed for user input
Lastly, a common misconception is that input data validation is only needed for user input fields such as forms or API requests. However, input data validation should also be applied to any data that enters the system, including data from external sources or database queries.
- External data can be manipulated or contain malicious content
- Database queries can have unexpected results if not properly validated
- Automated data imports or integrations should also undergo validation
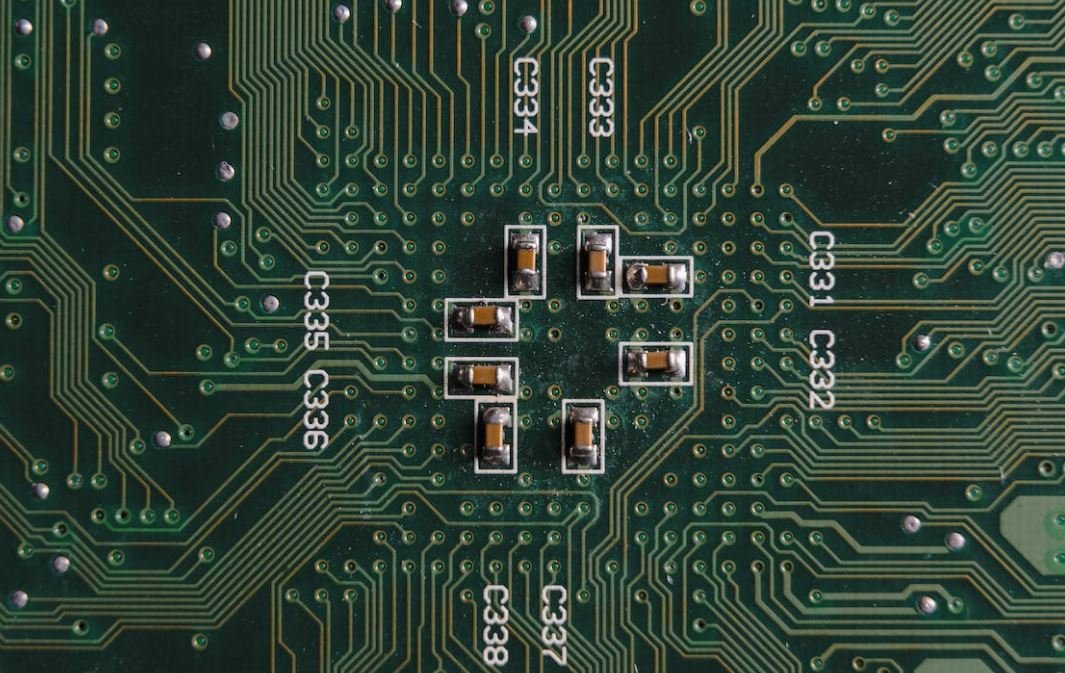
Introduction
Input data validation is an important aspect of any application to ensure the integrity and reliability of data. In Spring Boot, there are several mechanisms available for input data validation. This article provides an overview of these validation options along with real-life examples.
Data Validation Options in Spring Boot
Spring Boot offers various validation options to ensure that the input data meets specific criteria. These options can be applied at different levels within the application, such as at the entity level or during web requests. The following tables illustrate the different validation options available in Spring Boot and their associated properties.
Validation Annotations
Annotation | Description |
---|---|
@NotNull | Validates that the annotated element is not null. |
@NotEmpty | Validates that the annotated string is not null or empty. |
@Size | Validates that the size of the annotated element is within the specified bounds. |
Regular Expressions
Regex Pattern | Description |
---|---|
[A-Za-z]+ | Validates that the input contains only alphabetic characters. |
\d{4} | Validates that the input consists of exactly four digits. |
^[a-zA-Z0-9_]+$ | Validates that the input contains only alphanumeric characters and underscores. |
Custom Validators
In addition to the built-in validation annotations and regular expressions, Spring Boot allows us to create custom validators to validate input data according to specific rules. The following table showcases some examples of custom validators.
Validator | Description |
---|---|
EmailValidator | Validates that the input is a valid email address. |
AgeValidator | Validates that the input represents a valid age. |
PhoneNumberValidator | Validates that the input is a valid phone number. |
Validation Errors
When input data fails validation, Spring Boot provides detailed error messages to assist in identifying and resolving the issues. The following table displays some common validation error messages along with their descriptions.
Error | Description |
---|---|
Field ‘name’ must not be null | Indicates that the ‘name’ field of an entity must not be null. |
Field ’email’ must be a well-formed email address | Indicates that the ’email’ field of an entity must be in a valid email format. |
Field ‘age’ must be greater than or equal to 18 | Indicates that the ‘age’ field of an entity must be at least 18. |
Validation Groups
Group | Description |
---|---|
Creation | Validates the input data during entity creation. |
Update | Validates the input data during entity update. |
Deletion | Validates the input data during entity deletion. |
Bean Validation Constraints
Spring Boot leverages the Bean Validation framework to define constraints on input data. The following table showcases some commonly used constraints in Spring Boot applications.
Constraint | Description |
---|---|
@Pattern | Validates that the annotated element matches the regular expression pattern. |
@Min | Validates that the annotated element is greater than or equal to the specified minimum value. |
@Max | Validates that the annotated element is less than or equal to the specified maximum value. |
Conclusion
Input data validation plays a crucial role in maintaining data quality and preventing system vulnerabilities. In Spring Boot, developers have various options to validate input data, including built-in validation annotations, regular expressions, and custom validators. Additionally, Spring Boot provides clear error messages to troubleshoot validation failures. By incorporating input data validation mechanisms, developers can enhance the reliability and security of their applications.
Frequently Asked Questions
What is input data validation in Spring Boot?
Input data validation in Spring Boot refers to the process of validating and ensuring that the data received from users or external sources meets the specified criteria or constraints. It helps to prevent invalid or malicious data from being processed or stored, ensuring data integrity and security.
Why is input data validation important in Spring Boot?
Input data validation is crucial in Spring Boot applications to ensure the reliability, security, and consistency of the data. By validating input data, you can prevent common vulnerabilities like SQL injection, cross-site scripting (XSS), and other types of attacks.
How can I perform input data validation in Spring Boot?
In Spring Boot, you can perform input data validation by using various techniques. Some common approaches include using built-in validation annotations, implementing custom validators, and leveraging third-party libraries such as Hibernate Validator.
What are some built-in validation annotations in Spring Boot?
Spring Boot provides a set of built-in validation annotations that you can use to validate input data. Some commonly used annotations include @NotNull, @NotEmpty, @Size, @Min, @Max, @Pattern, and @Email.
Can I create custom validation annotations in Spring Boot?
Yes, you can create custom validation annotations in Spring Boot. By creating custom annotations, you can define your own validation rules and apply them to specific fields or objects in your application.
How can I handle validation errors in Spring Boot?
In Spring Boot, you can handle validation errors by using several approaches. One common approach is to use the @Valid annotation along with the BindingResult class to capture and process validation errors. Another approach is to use global exception handlers to handle validation errors in a centralized manner.
What is the role of Hibernate Validator in Spring Boot input data validation?
Hibernate Validator is a powerful validation framework that provides additional validation capabilities beyond the built-in annotations in Spring Boot. It allows you to define complex validation rules, implement custom validators, and integrate with Spring Boot seamlessly for input data validation.
Is input data validation performed on the client-side or server-side in Spring Boot?
In Spring Boot, input data validation is typically performed on the server-side. While client-side validation can provide a better user experience by catching errors before submitting the data to the server, server-side validation is essential for security and data integrity.
Can Spring Boot validate request parameters and query parameters?
Yes, Spring Boot can validate request parameters and query parameters. By using validation annotations on method parameters in your controller methods, you can validate the incoming request parameters and query parameters automatically.
Is input data validation enough to ensure application security in Spring Boot?
No, input data validation alone is not sufficient to ensure application security in Spring Boot. While input validation is an important security measure, it should be complemented with other security mechanisms such as authentication, authorization, data encryption, and secure coding practices to build a robust and secure application.