Input Data Type Python
Python is a versatile programming language that allows you to interact with different types of data. Understanding the input data type in Python is crucial for writing efficient and bug-free code. In this article, we will explore the various data types in Python and how they can be used.
Key Takeaways:
- Python supports several data types, including integers, floats, strings, lists, and dictionaries.
- Each data type has its own unique properties and methods.
- Understanding the input data type is essential for performing operations and type conversions accurately.
Integers are whole numbers in Python, such as 5, -10, or 1000. They can be used for mathematical calculations, indexing, and comparisons. *Integers are immutable in Python, meaning they cannot be changed after creation.*
Floats, also known as floating-point numbers, are decimal numbers in Python. These numbers are ideal for representing fractional and real values. *Floats are mutable and can be used to perform complex mathematical computations.*
Strings are sequences of characters enclosed in either single or double quotes. They are widely used to represent text data in Python. *Strings can be manipulated using various built-in string methods, making them highly versatile.*
Numeric Data Types in Python:
Data Type | Examples | Properties |
---|---|---|
Integer | 5, -10, 1000 | Immutable |
Float | 3.14, -2.5, 0.75 | Mutable |
Lists are ordered collections of items enclosed in square brackets. *They can contain elements of different data types and can be modified after creation.* Lists are useful for storing and manipulating multiple values in Python.
Data Type | Examples | Properties |
---|---|---|
String | ‘hello’, “world”, “123” | Versatile |
List | [1, ‘hello’, True] | Mutable |
Dictionaries are unordered collections of key-value pairs enclosed in curly braces. *They allow efficient lookup of values using unique keys.* Dictionaries are excellent for storing and accessing data in a structured manner.
Data Type | Examples | Properties |
---|---|---|
List | {‘name’: ‘John’, ‘age’: 25, ‘city’: ‘New York’} | Mutable |
Dictionary | {1: ‘apple’, 2: ‘banana’, 3: ‘orange’} | Versatile |
Understanding the input data type is essential for performing accurate operations and avoiding unexpected errors. By utilizing the appropriate data type, you can ensure your code executes smoothly and efficiently. So, next time you write Python code, consider the type of input data you are working with!
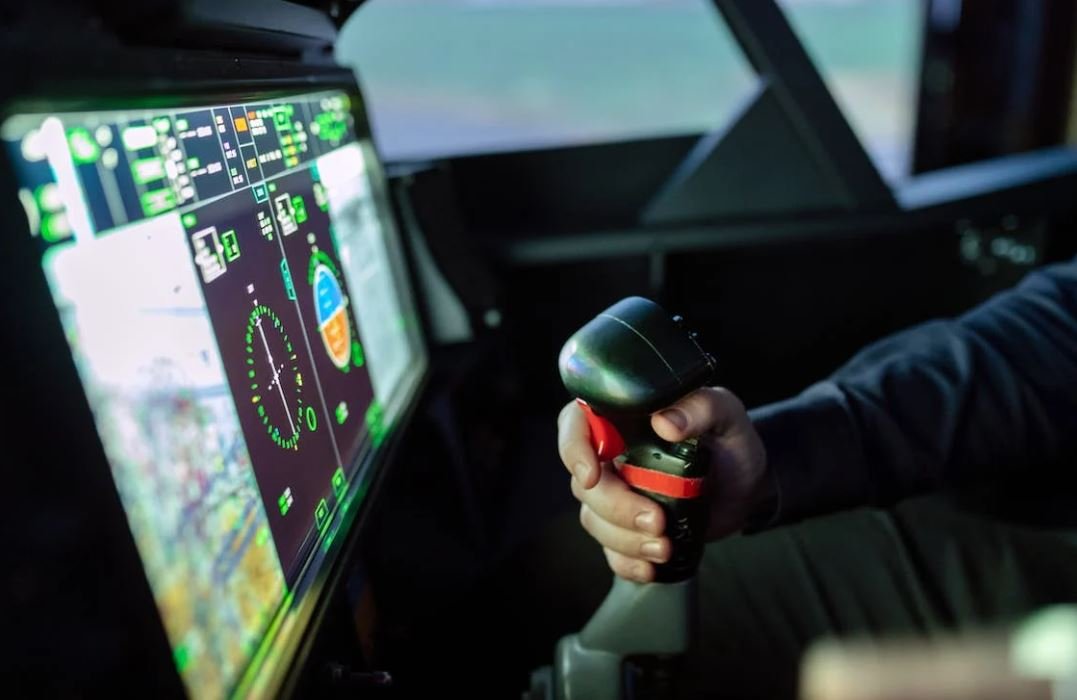
Common Misconceptions
1. Python does not support strong typing
One common misconception about Python is that it does not support strong typing. However, this is not true. Python is dynamically typed, meaning that the type of a variable is determined at runtime. While Python does allow for some flexibility with data types, it still enforces strict typing rules.
- Python has built-in support for type checking with annotations.
- Strong typing helps prevent common programming errors and improves code clarity.
- Python’s strict typing can be seen in its automatic type conversions and error handling.
2. All inputs entered in Python are treated as strings
Another misconception is that all inputs entered in Python are treated as strings. While it is true that user inputs are usually read as strings, Python provides functions to convert these inputs into appropriate data types. By utilizing functions such as int()
, float()
, or bool()
, the string inputs can be converted into integers, floats, or booleans respectively.
- Python’s
int()
function converts a string into an integer. - The
float()
function converts a string into a floating-point number. - The
bool()
function converts a string into a boolean value.
3. Python does not support type checking
Contrary to the belief that Python does not support type checking, it actually offers several tools for type checking and type hinting. With the addition of type annotations introduced in Python 3.5, developers can specify the expected type of variables and function return values. This allows for better code readability and facilitates catching type-related error at compile-time or by using external tools like mypy
.
- Type annotations were introduced in Python 3.5 to provide a way to declare the type of a variable.
- Python’s
mypy
tool can be used for static type checking. - Type hints can improve code documentation and can be used by IDEs for better autocompletion and static analysis.
4. Python does not have a dedicated input data type
It is often mistakenly assumed that Python does not have a dedicated input data type. However, in reality, Python does offer a data type specifically designed for receiving user input, called the input()
function. This function prompts the user for input and returns it as a string. Although the input is returned as a string, it can be converted into other data types depending on the requirements of the program.
- The
input()
function prompts the user to enter data. - The data entered through
input()
is always returned as a string. - The returned string can be converted into other data types using appropriate functions.
5. Python cannot handle user input errors
Finally, some people believe that Python cannot handle user input errors. While it is true that Python does not handle user input errors by default, developers can implement error handling mechanisms to handle invalid or unexpected input from users. By using techniques such as exception handling with try
and except
blocks, developers can gracefully handle input errors and prevent program crashes.
- Python’s exception handling can be used to catch and handle user input errors.
- The
try
andexcept
blocks can be used to specify error recovery code. - Handling input errors allows for a more robust and user-friendly experience.
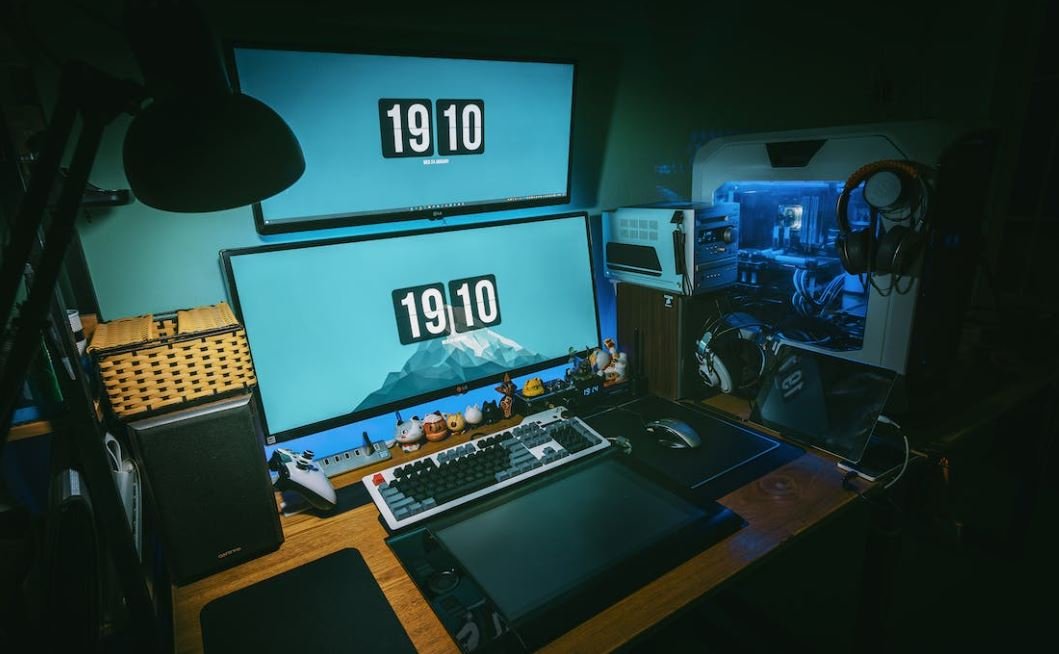
Python Versions and Release Dates
Python is a versatile programming language that has gone through various versions and updates throughout the years. Here is a table outlining some of the major Python versions and their respective release dates:
Version | Release Date |
---|---|
Python 1.0 | January 1994 |
Python 2.0 | October 2000 |
Python 2.7 | July 2010 |
Python 3.0 | December 2008 |
Python 3.6 | December 2016 |
Python 3.9 | October 2020 |
Popular Python Libraries and Their Usage
In addition to its core functionality, Python provides a vast collection of libraries that enhance its capabilities in various domains. Here are some well-known Python libraries and their common applications:
Library | Common Usage |
---|---|
Numpy | Scientific computing, array manipulation |
Pandas | Data analysis, manipulation, and visualization |
Matplotlib | Plotting graphs, charts, and figures |
Scikit-learn | Machine learning and data modeling |
Django | Web development and server-side programming |
Python Data Types and Examples
Python supports various data types that allow for flexible programming and manipulation of information. Here are some commonly used data types in Python:
Data Type | Example |
---|---|
Integer | 42 |
Float | 3.14 |
String | “Hello, Python!” |
List | [1, 2, 3] |
Dictionary | {“key”: “value”} |
Python Control Flow Statements
Control flow statements allow developers to control the order in which statements and blocks of code are executed. Here are some control flow statements in Python:
Statement | Description |
---|---|
if | Executes a block of code if a certain condition is true |
for | Iterates over a sequence of elements |
while | Executes a block of code as long as a certain condition is true |
break | Terminates the loop prematurely |
continue | Skips the rest of the current iteration and moves to the next one |
Python Built-in Functions
Python provides many built-in functions that simplify programming tasks. Here are some commonly used built-in functions in Python:
Function | Description |
---|---|
print() | Outputs text or variables to the console |
len() | Returns the length of an object |
range() | Generates a sequence of numbers |
max() | Returns the largest value from a sequence |
min() | Returns the smallest value from a sequence |
Python File Handling Modes
File handling is an essential aspect of many programming tasks. Python offers different modes for interacting with files. Here are some common file handling modes in Python:
Mode | Description |
---|---|
r | Reads a file (default mode) |
w | Writes to a file, creates a new file if it doesn’t exist, overwrites existing content |
a | Appends to a file, creates a new file if it doesn’t exist |
x | Creates a new file and opens it for writing |
t | Text mode (default mode) |
Python Exception Handling Keywords
Exception handling allows developers to handle and deal with errors or exceptional events that may occur during program execution. Here are some important exception handling keywords in Python:
Keyword | Description |
---|---|
try | Defines a block of code to be tested for exceptions |
except | Catches and handles specific exceptions |
finally | Defines a block of code to be executed, regardless of whether an exception occurs or not |
raise | Creates a custom exception and triggers it |
Python Regular Expression Metacharacters
Regular expressions are powerful tools for pattern matching and text manipulation. Python supports various metacharacters to refine search patterns. Here are some commonly used regular expression metacharacters:
Metacharacter | Description |
---|---|
. | Matches any single character except a newline |
^ | Matches the start of a string |
$ | Matches the end of a string |
* | Matches zero or more occurrences of the preceding character or group |
+ | Matches one or more occurrences of the preceding character or group |
Python Database Connectivity Libraries
Python provides several libraries to connect and interact with databases, enabling seamless integration with data storage and retrieval. Here are some popular Python libraries for database connectivity:
Library | Description |
---|---|
SQLite3 | Python interface to the SQLite database engine |
psycopg2 | PostgreSQL adapter for Python |
mysql-connector-python | MySQL driver for Python |
pyodbc | Python library for ODBC (Open Database Connectivity) |
cx_Oracle | Python interface for Oracle Database |
In conclusion, Python is a versatile language with a rich ecosystem. Its numerous versions, powerful libraries, flexible data types, and various control flow statements enable developers to tackle diverse programming tasks. Additionally, Python’s built-in functions, file handling modes, exception handling keywords, regular expression metacharacters, and database connectivity libraries contribute to its extensive functionality. With its widespread adoption and continuous evolution, Python remains a vital tool for developers across industries.
Frequently Asked Questions
What are the different data types in Python?
The different data types in Python include:
- Integer
- Float
- String
- List
- Tuple
- Dictionary
- Boolean
- Set
How do I determine the data type of a variable in Python?
You can determine the data type of a variable using the type()
function. For example, if you have a variable named my_var
, you can determine its data type by calling type(my_var)
.
What is type conversion in Python?
Type conversion, also known as type casting, is the process of converting one data type to another. Python provides several built-in functions to perform type conversion, such as int()
, float()
, str()
, etc.
Can you give an example of type conversion in Python?
Sure! Here’s an example:
x = 10 # Integer
y = str(x) # Converted to string
z = float(x) # Converted to float
What is the difference between a list and a tuple in Python?
A list is a mutable data type, which means its elements can be changed. On the other hand, a tuple is an immutable data type, where its elements cannot be modified once defined.
How do I access elements in a list or a tuple in Python?
You can access elements in a list or a tuple using indexing. For example, to access the first element, you can use my_list[0]
or my_tuple[0]
.
What is a dictionary in Python?
A dictionary is an unordered collection of key-value pairs. Each value in a dictionary is associated with a unique key, which allows for efficient retrieval of values based on keys.
How do I add or modify elements in a dictionary?
To add or modify elements in a dictionary, you can assign a value to a new key or an existing key. For example:
my_dict = {"name": "John", "age": 25}
my_dict["gender"] = "Male" # Adding a new key-value pair
my_dict["age"] = 26 # Modifying an existing value
What is a boolean data type in Python?
A boolean data type represents one of two values: True
or False
. It is often used for logical operations and conditional statements.
What is a set in Python?
A set is an unordered collection of unique elements. It is useful for operations that require membership testing and eliminating duplicate values.