How to Learn Computer Algorithms
Computer algorithms are the backbone of modern technology, powering everything from search engines and social media platforms to autonomous vehicles and artificial intelligence systems. Learning algorithms can be intimidating, but with the right approach and resources, anyone can grasp the fundamentals. In this article, we will provide you with a step-by-step guide on how to learn computer algorithms effectively.
Key Takeaways:
- Understanding the importance and applications of computer algorithms.
- Breaking down complex algorithms into smaller, manageable parts.
- Practicing algorithmic problem-solving through coding challenges and exercises.
- Utilizing online resources and communities for learning and support.
- Continuously improving and staying up-to-date with algorithmic techniques and strategies.
1. Understand the Importance of Algorithms
Before diving into learning algorithms, it’s essential to grasp their significance. Algorithms are step-by-step instructions or procedures designed to solve specific computational problems. They are the building blocks that enable computers to process, analyze, and make decisions efficiently. *Mastering algorithms allows you to optimize performance, solve complex problems, and think critically.*
2. Break Down Complex Algorithms
Complex algorithms can seem overwhelming at first. The key is to break them down into smaller, more manageable parts. Start by understanding the core concepts, such as data structures, search, sort, and recursion. Then progressively delve deeper into more advanced algorithms, such as graph traversal or dynamic programming. *Taking small steps to comprehend each component will gradually lead to a comprehensive understanding of complex algorithms.*
3. Practice Algorithmic Problem-Solving
Practicing algorithmic problem-solving is crucial to enhance your algorithmic thinking skills. Solve coding challenges and exercises that focus on algorithms and data structures. Websites like LeetCode, HackerRank, and CodeSignal offer a multitude of algorithmic problems to solve. *By consistently practicing problem-solving, you will become more adept at identifying patterns and developing efficient algorithms.*
4. Utilize Online Resources and Communities
Take advantage of the vast array of online resources and communities dedicated to algorithms. Online platforms like Coursera, edX, and Khan Academy offer comprehensive algorithm courses. Connect with like-minded individuals by joining algorithm-focused forums or communities like Stack Overflow or Reddit’s r/algorithms. *Collaborating and seeking guidance from others can greatly enhance your learning journey.*
5. Continuously Improve and Stay Up-to-Date
Algorithms evolve rapidly, and it’s crucial to stay up-to-date with the latest techniques and strategies. Read algorithm books, research papers, and follow algorithm news to expand your knowledge. *Embracing a growth mindset and consistently seeking improvement will help you stay ahead in the field of computer algorithms.*
Data and Statistics:
Table 1: Algorithmic Efficiency Comparison | |
---|---|
Algorithm | Time Complexity |
Bubble Sort | O(n^2) |
Merge Sort | O(n log n) |
Quicksort | O(n log n) |
Table 2: Common Data Structures | ||
---|---|---|
Data Structure | Description | Time Complexity |
Array | A linear collection of elements. | O(1) (access), O(n) (search/insert/delete) |
Linked List | A data structure where each element contains a reference to the next element. | O(n) (access/search), O(1) (insert/delete) |
Hash Table | A data structure that stores key-value pairs in a hash function-based structure. | O(1) (search/insert/delete in average case) |
Table 3: Algorithmic Problems and Solutions | ||
---|---|---|
Problem | Algorithm | Solution |
Two Sum | Hash Table | Iterate over the array, check if the target minus the current element exists in the hash table. |
Longest Increasing Subsequence | Dynamic Programming | Maintain a dynamic programming array to store the length of the longest increasing subsequence. |
Merge Intervals | Sorting | Sort the intervals by start time, then merge overlapping intervals. |
By following these steps and leveraging available resources, you can develop a solid foundation in computer algorithms and enhance your problem-solving skills. Remember, the journey of learning algorithms is continuous, and staying updated with new techniques and strategies will be paramount to your success in the field.

Common Misconceptions
Misconception: Algorithms are only for advanced programmers
Many people believe that algorithms are complex concepts that can only be understood by experienced programmers. However, this is far from the truth.
- Algorithms can be learned by anyone, regardless of their programming experience.
- There are beginner-friendly resources available that provide a step-by-step approach to learning algorithms.
- Understanding algorithms can enhance problem-solving skills for individuals at any level of programming knowledge.
Misconception: Algorithms are only used in academia or research
Another common misconception is that algorithms have limited real-world applications and are only used in academic or research environments.
- Algorithms are used extensively in industries such as finance, healthcare, and technology.
- They play a crucial role in optimizing processes, sorting data, and making efficient decisions in various applications.
- By understanding algorithms, individuals can improve their coding abilities and develop more efficient and effective solutions to everyday problems.
Misconception: Memorization is the key to mastering algorithms
Some people believe that mastering algorithms requires memorizing complex formulas and use cases. However, this is not the case.
- Understanding the underlying logic and core principles is more important than memorization.
- Practicing by solving algorithmic problems is essential for improving algorithmic thinking.
- Applying problem-solving strategies and analyzing different algorithms can enhance comprehension and enable individuals to come up with optimized solutions.
Misconception: Algorithms are always the most optimal solution
It is a common misconception that algorithms always provide the most optimal solution for a given problem.
- Choosing the right algorithm depends on various factors, such as the size of the input, time constraints, and desired outcomes.
- There can be multiple valid algorithms to solve a problem, each with its own advantages and trade-offs.
- Understanding algorithmic complexity and analyzing different algorithms can help individuals select the most suitable solution for a specific problem.
Misconception: Learning algorithms is a one-time task
Some people mistakenly think that once they learn a few algorithms, they have acquired all the necessary knowledge in this field.
- Algorithmic learning is an ongoing process due to the evolving nature of technology and problem domains.
- New algorithms and optimization techniques are constantly being developed, requiring professionals to continuously update their knowledge.
- Regular practice and exposure to different algorithms are crucial for strengthening algorithmic skills and staying up to date with advancements in the field.
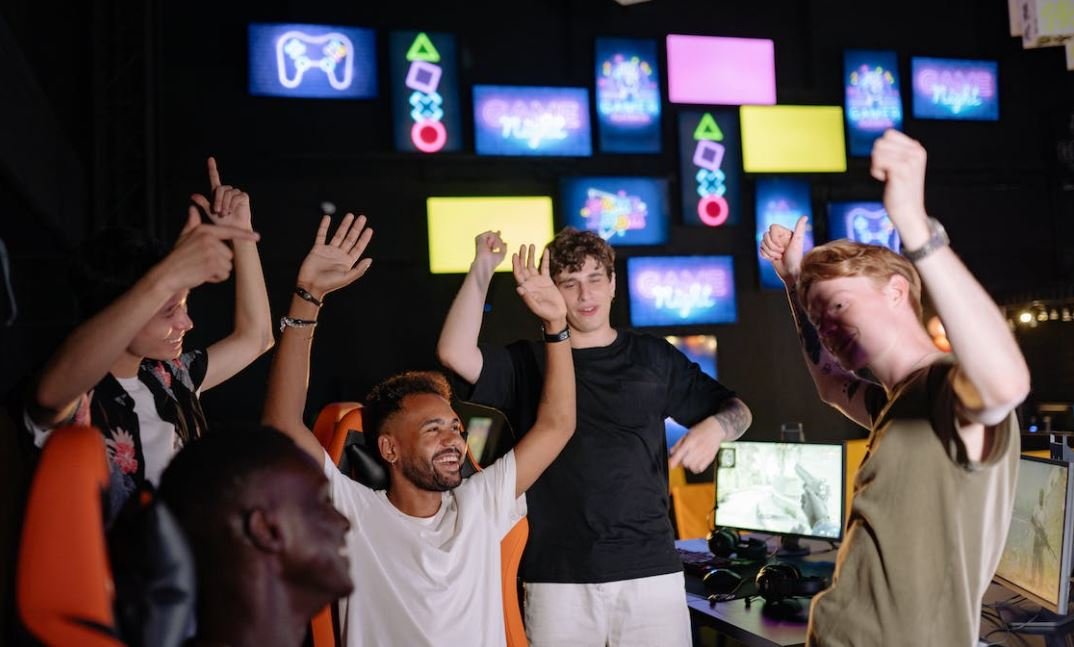
Introduction to Computer Algorithms
Computer algorithms are essential for solving complex computational problems efficiently. They provide a set of instructions or rules for executing a task step-by-step. Learning computer algorithms is crucial for anyone interested in programming, data analysis, or problem-solving. The following tables provide some interesting insights and examples related to computer algorithms.
Table: Time Complexity of Common Algorithms
The time complexity of an algorithm measures the amount of time it takes to run as a function of the input size. Here is a comparison of the time complexities for some commonly used algorithms:
Algorithm | Time Complexity |
---|---|
Linear Search | O(n) |
Binary Search | O(log n) |
Bubble Sort | O(n^2) |
Merge Sort | O(n log n) |
Table: Sorting Algorithms and Their Best Use Cases
Sorting algorithms are fundamental in computer science and data processing. Different sorting algorithms have varying efficiency and suitability for different scenarios. The table below illustrates some sorting algorithms and their best use cases:
Sorting Algorithm | Best Use Cases |
---|---|
Bubble Sort | Small-sized arrays |
Insertion Sort | Partially sorted arrays |
Quick Sort | Large-sized arrays |
Merge Sort | Linked lists, large arrays, external sorting |
Table: Comparison of Different Search Algorithms
When searching for specific elements in a collection of data, different search algorithms can be used. Here is a comparison of some common search algorithms:
Search Algorithm | Complexity | Best Use Case |
---|---|---|
Linear Search | O(n) | Unsorted data |
Binary Search | O(log n) | Sorted data |
Hash Table | O(1) | Key-value pairs, quick access |
Table: Comparison of Space Complexity
Space complexity refers to the amount of memory or space an algorithm requires to solve a problem. The table below compares the space complexities of different algorithms:
Algorithm | Space Complexity |
---|---|
Bubble Sort | O(1) |
Quick Sort | O(log n) |
Merge Sort | O(n) |
Table: Real-life Applications of Algorithms
Algorithms are not just theoretical concepts; they find applications in various fields. The table below highlights some real-life examples where algorithms play a crucial role:
Field | Application |
---|---|
Internet | PageRank algorithm for search engine results |
Transportation | Shortest path algorithms for navigation systems |
Finance | Algorithms for stock market analysis |
Healthcare | Medical image analysis algorithms |
Table: Famous Algorithms and Their Inventors
Throughout history, brilliant minds have developed groundbreaking algorithms. Here are some famous algorithms and the individuals behind them:
Algorithm | Inventor(s) |
---|---|
QuickSort | Tony Hoare |
Dijkstra’s Algorithm | Edsger Dijkstra |
PageRank | Larry Page, Sergey Brin |
Table: Biggest Challenges in Algorithm Design
Designing efficient algorithms often comes with certain challenges. This table highlights some significant challenges faced by algorithm designers:
Challenge | Description |
---|---|
Time Complexity | Finding the optimal balance between speed and accuracy |
Optimization | Minimizing resources required while maintaining efficiency |
Scalability | Ensuring algorithms work efficiently with large datasets |
Table: Complexity Classes and Examples
Complexity classes categorize problems based on their computational complexity. Here are some commonly known complexity classes and examples of problems they represent:
Complexity Class | Problem Example |
---|---|
P | Linear programming |
NP-hard | Traveling Salesman Problem |
NP-complete | Boolean satisfiability problem |
Conclusion
Learning computer algorithms is a fundamental aspect of becoming a proficient programmer or problem-solver. Through the tables presented, we can gain insights into algorithmic complexities, suitable use cases, real-world applications, and the challenges involved in algorithm design. By understanding these concepts, we can optimize our code, enhance efficiency, and solve complex problems effectively.
Frequently Asked Questions
What is a computer algorithm?
An algorithm is a step-by-step procedure to solve a specific problem or accomplish a particular task using computational steps.
Why is it important to learn computer algorithms?
Learning computer algorithms is crucial for developing efficient and optimized software solutions. Algorithms are at the core of computer science and help in problem-solving and designing efficient programs.
How can beginners start learning computer algorithms?
Beginners can start learning computer algorithms by understanding the basic concepts, data structures, and algorithmic design techniques. They can refer to online tutorials, books, or take online courses specifically designed for algorithmic learning.
What are some popular algorithm design techniques?
Some popular algorithm design techniques include divide and conquer, dynamic programming, greedy algorithms, backtracking, and more. Each technique has its own advantages and is suitable for solving specific types of problems.
What are data structures, and why are they important in algorithms?
Data structures are ways of organizing and storing large amounts of data. They play a crucial role in algorithms as the choice of data structure affects the efficiency and time complexity of algorithms.
What are some common examples of computer algorithms?
Some common examples of computer algorithms include sorting algorithms like bubble sort, merge sort, search algorithms like linear search, binary search, and graph algorithms like Dijkstra’s algorithm, BFS, DFS.
How can one improve their algorithmic skills?
Improving algorithmic skills requires practice. Solving algorithmic problems, participating in programming competitions, and analyzing efficient algorithms can help in enhancing algorithmic skills.
How can algorithms be analyzed for efficiency?
Algorithms can be analyzed for efficiency by calculating their time complexity and space complexity. Time complexity measures the amount of time required for an algorithm to run, while space complexity determines the amount of memory required.
What are some resources for learning computer algorithms?
Some resources for learning computer algorithms include online coding platforms like LeetCode, Codecademy, Coursera, online tutorials, books such as “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein, and educational websites like GeeksforGeeks and Khan Academy.
Are there any prerequisites for learning computer algorithms?
While not mandatory, having a basic understanding of programming concepts and data structures is useful when learning computer algorithms. Familiarity with a programming language like Python, Java, or C++ can also be beneficial.