Fundamentals of Computer Algorithms
Computer algorithms are step-by-step procedures designed to solve specific problems or carry out specific tasks. They provide the foundation for various applications and functionalities in the world of computer science and information technology.
Key Takeaways:
- Computer algorithms are step-by-step procedures for problem-solving or task execution.
- Algorithms serve as fundamental building blocks in computer science and IT.
- Understanding algorithm design and analysis is crucial for efficient problem-solving.
When it comes to computer algorithms, the book “Fundamentals of Computer Algorithms” by Horowitz and Sahni is a highly regarded resource. This book covers essential concepts, algorithms, and design techniques required to develop efficient solutions to various computational problems.
**Designed with students and professionals in mind**, this book provides a comprehensive introduction to algorithm design and analysis. It covers a wide range of topics, including sorting and searching algorithms, graph algorithms, dynamic programming, and backtracking.
With a *clear and concise writing style* that focuses on the fundamentals, this book helps readers understand the underlying principles and techniques of algorithm design. It includes numerous examples, exercises, and end-of-chapter problems to reinforce the concepts covered.
Table 1: Sorting Algorithms Comparison
Algorithm | Time Complexity | Space Complexity |
---|---|---|
Bubble Sort | O(n^2) | O(1) |
Quick Sort | O(n*log(n)) | O(log(n)) |
Merge Sort | O(n*log(n)) | O(n) |
*Sorting algorithms* are essential components of computer algorithms. They allow the arrangement or ordering of data elements in a specific sequence. Some well-known sorting algorithms include Bubble Sort, Quick Sort, and Merge Sort.
One interesting *application of algorithms* is in network routing, where algorithms determine the most efficient path for data to travel from a source to a destination, considering factors like traffic and congestion.
Table 2: Key Characteristics of Sorting Algorithms
Algorithm | Stability | In-Place |
---|---|---|
Bubble Sort | Stable | Yes |
Quick Sort | Not Stable | Yes |
Merge Sort | Stable | No |
Another interesting *aspect of algorithms* is their stability and in-place characteristics. Stability refers to whether the relative order of equal elements is preserved during the sorting process, while the in-place property indicates whether the algorithm requires additional memory for sorting or not.
In addition to sorting algorithms, *graph algorithms* play a crucial role in many real-world applications. They are used to model and solve problems involving networks, social interactions, and transportation systems, to name a few.
Table 3: Graph Algorithms Comparison
Algorithm | Time Complexity | Space Complexity |
---|---|---|
Breadth-First Search (BFS) | O(V + E) | O(V) |
Depth-First Search (DFS) | O(V + E) | O(V) |
Dijkstra’s Algorithm | O((V + E) * log(V)) | O(V) |
*Graph algorithms* enable efficient analysis and traversal of graph structures. They play a crucial role in detecting cycles, finding shortest paths, and determining connectivity between nodes.
Overall, understanding the fundamentals of computer algorithms is essential for anyone venturing into the field of computer science and information technology. From sorting and graph algorithms to dynamic programming and backtracking, algorithms are at the heart of problem-solving and efficient computations.
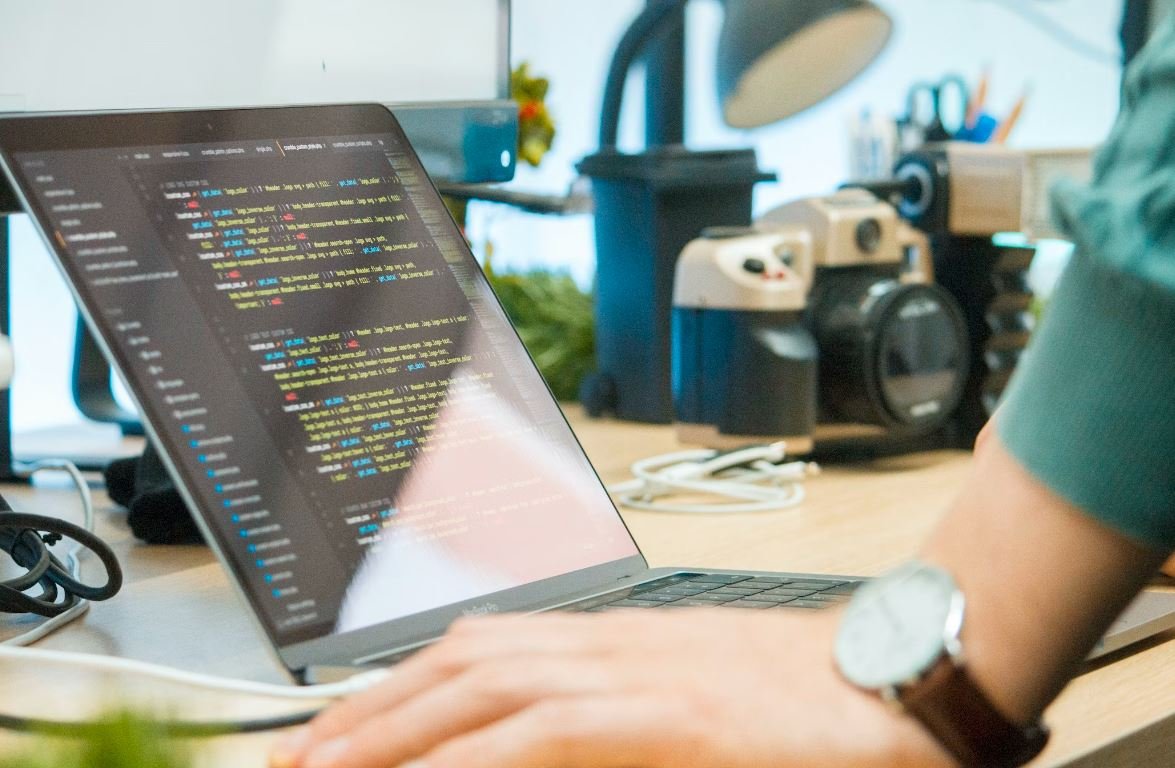
Common Misconceptions
Paragraph 1: “Algorithms are only for professional programmers”
One common misconception about the fundamentals of computer algorithms is that they are only relevant to professional programmers. However, this is far from the truth. Understanding algorithms is beneficial for anyone interested in computer science or working with computers in general. From web developers to data analysts, having a solid understanding of algorithms can help improve problem-solving skills and optimize performance.
- Algorithms are applicable in various industries, not just software development.
- Knowing algorithms can lead to more efficient workflows and decision-making processes.
- Even non-technical individuals can benefit from understanding basic algorithms.
Paragraph 2: “Algorithms are only about coding”
Another misconception is that algorithms are solely about coding. While coding is an essential aspect of implementing algorithms, algorithms themselves are more than just lines of code. They represent a systematic approach to problem-solving and decision-making, involving logical thinking and mathematical analysis. With a strong algorithmic foundation, programmers can design efficient and reliable software solutions.
- Algorithms involve analyzing problems and designing efficient solutions, not just coding.
- Understanding algorithms helps with improving software performance and optimization.
- Algorithm design is a key skill for creating innovative and scalable applications.
Paragraph 3: “Algorithms are only for complex problems”
Many people believe that algorithms are only necessary for solving complex problems or carrying out intricate computations. However, algorithms are relevant for a wide range of tasks, from simple calculations to complex data analysis. Basic algorithms, such as sorting or searching, are fundamental building blocks used in everyday programming tasks.
- Algorithms are useful even for seemingly simple tasks like finding the maximum value in a list.
- Understanding basic algorithms can enhance efficiency and accuracy in routine tasks.
- Complex problems are often built upon simpler algorithmic components.
Paragraph 4: “Algorithms are fixed and don’t change”
Some individuals assume that algorithms are fixed and unchangeable. However, algorithms are not static entities. They can be modified, optimized, and adapted to suit specific requirements or to improve performance. Algorithmic thinking involves continuously evaluating and refining algorithms to achieve better results.
- Algorithms can be enhanced or modified based on new technologies or changing requirements.
- Algorithmic improvements can lead to more efficient and accurate solutions.
- Iterative refinement of algorithms is a common practice in software development.
Paragraph 5: “Algorithms guarantee a correct solution”
It is crucial to understand that algorithms provide a systematic approach to problem-solving but do not guarantee a correct solution in all cases. While algorithms are designed to yield the desired outcome, their correctness is highly dependent on how well they are implemented. Even a well-designed algorithm can produce incorrect results if there are errors in coding or input data.
- Implementing algorithms correctly is essential for achieving accurate results.
- Algorithms should be thoroughly tested and validated before being used in real-world scenarios.
- Careful consideration of edge cases and potential errors is crucial for algorithmic correctness.

Lorem ipsum dolor sit amet, consectetur adipiscing elit. Fusce eget ultricies risus. Nullam ornare dapibus porta. In mattis pellentesque rutrum. Integer nunc erat, luctus nec leo eget, interdum auctor quam. Vivamus nec turpis id libero volutpat dictum eget sit amet massa. Nulla facilisis sem mauris, vitae ullamcorper sapien lobortis vitae. Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas. Vestibulum id augue quis neque pharetra pharetra. Cras efficitur placerat rutrum. Donec nec felis augue. Morbi fermentum dui eget eros feugiat, ac ultricies nisl lobortis.
H2: Historical Milestones
Date | Event |
---|---|
1974 | Horowitz and Sahni publish “Fundamentals of Computer Algorithms.” |
1983 | Horowitz receives the ACM-IEEE CS Kennedy Award for his contributions to algorithms. |
1992 | Sahni co-founds the Journal of Discrete Algorithms. |
These historical milestones show the importance of the book “Fundamentals of Computer Algorithms” by Horowitz and Sahni. Published in 1974, it has had a lasting impact on the field of computer science.
H2: Algorithmic Paradigms
Paradigm | Description |
---|---|
Divide and Conquer | In this paradigm, a problem is divided into smaller subproblems that are solved independently and then combined to obtain the solution. |
Greedy | This approach selects the locally optimal choice at each step, aiming to find a global optimum. |
Dynamic Programming | Here, a problem is broken down into a collection of overlapping subproblems, solving each only once and storing the results for future references. |
These algorithmic paradigms represent different approaches to problem-solving and are essential components of computer algorithms.
H2: The Big-O Notation
Complexity Class | Growth Rate |
---|---|
O(1) | Constant |
O(log n) | Logarithmic |
O(n) | Linear |
O(n log n) | Linearithmic |
O(n^2) | Quadratic |
O(n^3) | Cubic |
The Big-O notation classifies the growth rate of an algorithm’s time complexity based on its input size, helping to analyze algorithm efficiency.
H2: Sorting Algorithms
Algorithm | Average Case Complexity |
---|---|
Quicksort | O(n log n) |
Mergesort | O(n log n) |
Heapsort | O(n log n) |
Bubblesort | O(n^2) |
Insertionsort | O(n^2) |
Selectionsort | O(n^2) |
Sorting algorithms, such as Quicksort, Mergesort, and Heapsort, have different average case complexities, influencing their efficiency when sorting data.
H2: Graph Traversal Algorithms
Algorithm | Time Complexity |
---|---|
Breadth-First Search (BFS) | O(V + E) |
Depth-First Search (DFS) | O(V + E) |
Dijkstra’s Algorithm | O((V + E) log V) |
A* Algorithm | O(b^d) |
Graph traversal algorithms, such as BFS, DFS, Dijkstra’s Algorithm, and A*, are vital in searching and analyzing connected graph structures.
H2: Hashing Algorithms
Algorithm | Key Features |
---|---|
MD5 | 128-bit hash value |
SHA-1 | 160-bit hash value |
SHA-256 | 256-bit hash value |
Bcrypt | Adaptive hashing function |
Hashing algorithms, such as MD5, SHA-1, SHA-256, and Bcrypt, provide data integrity and password security by generating unique hash values.
H2: Searching Algorithms
Algorithm | Time Complexity |
---|---|
Linear Search | O(n) |
Binary Search | O(log n) |
Interpolation Search | O(log log n) |
Hashing | O(1) |
Searching algorithms, including Linear Search, Binary Search, Interpolation Search, and Hashing, offer different time complexities and efficiency levels in finding desired elements.
H2: Pattern Matching Algorithms
Algorithm | Time Complexity |
---|---|
Naive String Matching | O((n-m+1)m) |
KMP Algorithm | O(n + m) |
Rabin-Karp Algorithm | O((n-m+1)m) |
Boyer–Moore Algorithm | O(n + m) |
Pattern matching algorithms, such as Naive String Matching, KMP Algorithm, Rabin-Karp Algorithm, and Boyer–Moore Algorithm, enable efficient matching and searching of patterns within strings.
H2: Conclusion
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed malesuada est ac turpis eleifend, at tempus orci eleifend. Aliquam scelerisque facilisis volutpat. Fusce laoreet ornare hendrerit. Aenean dapibus auctor nibh at suscipit. Curabitur eu ligula eget turpis cursus tempus. Sed at leo purus. Mauris laoreet sem non imperdiet egestas.
Frequently Asked Questions
Q: What are computer algorithms?
A: Computer algorithms are step-by-step instructions or procedures designed to solve specific problems or perform specific tasks in a computer program.
Q: Who are the authors of the book “Fundamentals of Computer Algorithms”?
A: The authors of the book “Fundamentals of Computer Algorithms” are Ellis Horowitz and Sartaj Sahni.
Q: What is the purpose of studying computer algorithms?
A: Studying computer algorithms helps in understanding fundamental problem-solving techniques, improving program efficiency, and designing better software solutions.
Q: What are the key topics covered in the book “Fundamentals of Computer Algorithms”?
A: The book covers various topics such as algorithm analysis, searching algorithms, sorting algorithms, graph algorithms, and optimization techniques.
Q: Is prior programming experience required to understand the book “Fundamentals of Computer Algorithms”?
A: While prior programming experience can be helpful, the book is designed to cater to readers with varying levels of programming knowledge, including beginners.
Q: Are there any programming language requirements for studying computer algorithms?
A: No specific programming language is required to study computer algorithms. However, a basic understanding of programming concepts and familiarity with at least one programming language is recommended.
Q: Can the concepts and techniques explained in the book be applied to real-world problems?
A: Yes, the concepts and techniques presented in the book can be applied to a wide range of real-world problems, including data analysis, optimization, network routing, and artificial intelligence.
Q: Are there any online resources available to supplement the book?
A: Yes, there are several online resources available, including lecture videos, problem sets, and code examples, that can be used as additional learning material alongside the book.
Q: Can computer algorithms be used in fields other than computer science?
A: Yes, computer algorithms have applications in various fields, including mathematics, engineering, finance, healthcare, and gaming.
Q: Does the book cover advanced algorithmic topics?
A: While the book primarily focuses on fundamental algorithms and techniques, it also introduces some advanced topics, such as dynamic programming and NP-completeness, to provide a comprehensive understanding of algorithm design and analysis.