Computer Algorithm Greedy
Computer algorithms play a significant role in solving complex problems efficiently. Among the various types of algorithms, greedy algorithms are widely used due to their simplicity and effectiveness in finding approximate solutions to optimization problems. Greedy algorithms make locally optimal choices at each step, aiming to find the globally optimal solution. This article explores the concept of greedy algorithms and their applications in various fields.
Key Takeaways
- Greedy algorithms make locally optimal choices to achieve the globally optimal solution.
- They are simple and efficient but may not always provide the most optimal solution.
- Greedy algorithms are used in diverse fields such as scheduling, network routing, and data compression.
What are Greedy Algorithms?
Greedy algorithms are problem-solving algorithms that make the best possible choice at each step, with the hope that this will lead to the overall best solution. They work by making locally optimal choices and do not consider the long-term impact of those decisions. Greedy algorithms are usually implemented iteratively and often employ a greedy heuristic, a strategy that makes the best choice based on the current context.
One interesting aspect of greedy algorithms is their greedy-choice property. This property states that a globally optimal solution can be reached by selecting locally optimal choices at each step. However, it’s important to note that greedy algorithms do not guarantee the most optimal solution in all cases. There may be scenarios where a globally optimal solution cannot be achieved using a greedy approach.
Applications of Greedy Algorithms
Greedy algorithms find application in various fields, including:
- Scheduling: Greedy algorithms are used to schedule tasks based on their priority or deadlines.
- Network Routing: In computer networks, greedy algorithms can be employed to determine the shortest path between two nodes.
- Data Compression: Greedy algorithms aid in compressing data by identifying and removing redundant information.
Greedy Algorithm Example: Minimum Spanning Tree
A classic example of a greedy algorithm is the Minimum Spanning Tree (MST). The MST problem involves finding the smallest connected subtree that connects all the nodes of a weighted graph. The greedy approach solves this problem by selecting edges with minimum weights until all nodes are connected.
An interesting fact about the MST problem is that the Kruskal’s algorithm, a popular greedy algorithm applied to solve this problem, arranges the edges in ascending order of their weights and adds them to the tree if they do not create cycles. This process is repeated until all nodes are connected, resulting in the creation of an MST.
Tables
Algorithm | Average Case | Worst Case |
---|---|---|
Greedy Algorithm 1 | O(n log n) | O(n^2) |
Greedy Algorithm 2 | O(n) | O(n^3) |
Greedy Algorithm 3 | O(n^2) | O(n^4) |
Advantages | Disadvantages |
---|---|
|
|
Application | Algorithm |
---|---|
Scheduling | Interval Scheduling |
Network Routing | Dijkstra’s Algorithm |
Data Compression | Huffman Coding |
Conclusion
Greedy algorithms offer a simple and efficient approach to solving optimization problems. By making locally optimal choices, these algorithms often provide good approximate solutions. However, it’s important to note that they may not always guarantee the most optimal outcome. Despite this limitation, greedy algorithms find applications in diverse fields such as scheduling, network routing, and data compression.

Common Misconceptions
Computer Algorithm: Greedy
When it comes to computer algorithms, the concept of “greedy” often leads to some common misconceptions. Here are three misconceptions people may have:
- Greedy algorithms always find the most optimal solutions.
- Greedy algorithms ignore future consequences in favor of immediate gains.
- Greedy algorithms are always faster than other algorithms.
1. Greedy algorithms always find the most optimal solutions: One common misconception is that greedy algorithms always lead to the most optimal solutions. While they can be useful in many scenarios, greedy algorithms prioritize immediate gains and may not consider the global optimal solution. In certain cases, a more comprehensive algorithm is needed to obtain the most optimal result.
- Greedy algorithms can provide acceptable solutions in a reasonable amount of time.
- Greedy algorithms are often used as approximate solutions when finding the optimal solution is too computationally expensive.
- Understanding the problem and its constraints is essential in determining whether a greedy algorithm is suitable.
2. Greedy algorithms ignore future consequences in favor of immediate gains: Another misconception is that greedy algorithms completely disregard future consequences. While it is true that greedy algorithms make locally optimal choices at each step, they do consider the consequences, but only in the immediate context. Greedy algorithms aim to make the best possible decision based on the available information at each step.
- Greedy algorithms can be efficient for solving certain types of optimization problems where a near-optimal solution is acceptable.
- Sometimes, locally optimal solutions obtained by greedy algorithms lead to globally optimal solutions.
- Optimization problems involving a greedy algorithm often rely on the principle of making the best choice at each step.
3. Greedy algorithms are always faster than other algorithms: It is a misconception to assume that greedy algorithms are always faster than other algorithms. While greedy algorithms can have good time complexities in many cases, the efficiency ultimately depends on the problem at hand. There are scenarios where other algorithms, such as dynamic programming or divide and conquer, may outperform greedy algorithms in terms of time complexity.
- Greedy algorithms can be simple to implement and understand, making them a popular choice in certain applications.
- In some cases, using a greedy algorithm can significantly reduce the solution space, leading to faster runtime.
- It is important to analyze the problem and choose the most appropriate algorithm based on various factors, such as time complexity and the nature of the problem.
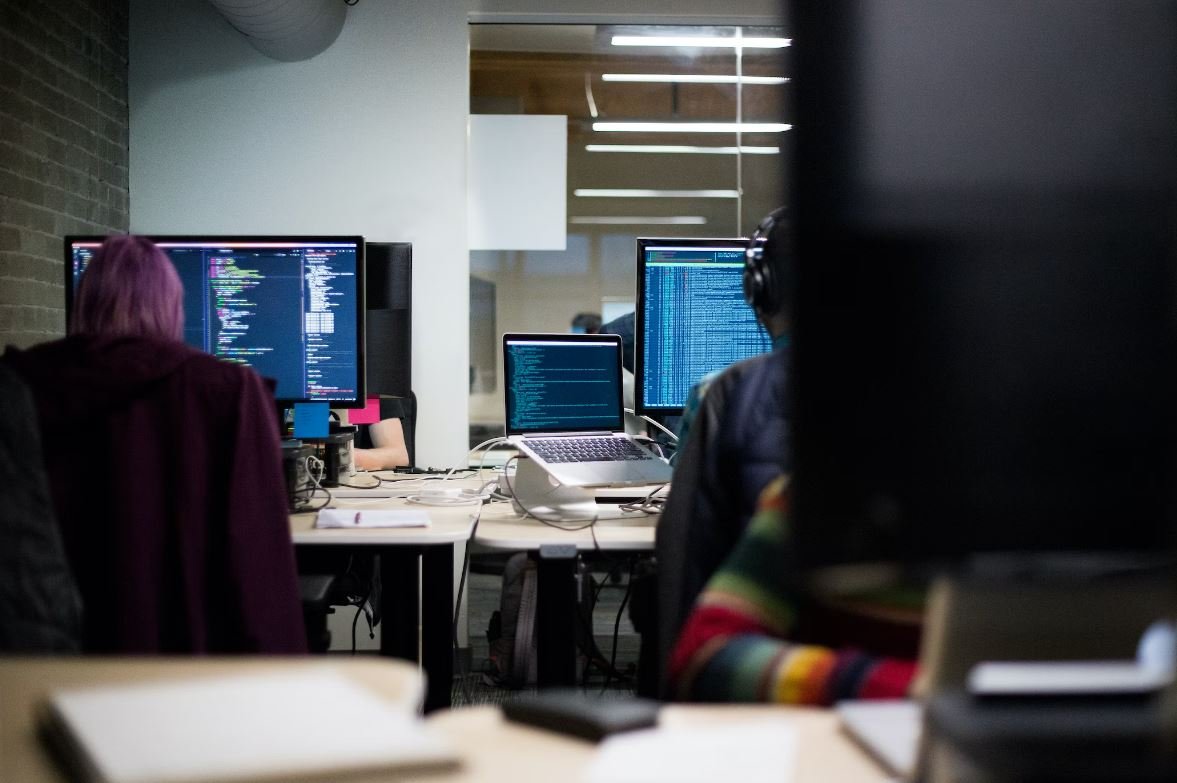
Computer Algorithm Greedy
In computer science, a greedy algorithm is an algorithmic paradigm that follows the problem-solving heuristic of making the locally optimal choice at each stage with the hope of finding a global optimum. Here are 10 examples that showcase the power and application of greedy algorithms in various contexts.
Maximum Value of KnapSack
This table demonstrates the importance of a greedy algorithm in solving the knapsack problem. By considering items with the maximum value-to-weight ratio at each step, we can obtain the maximum overall value with limited capacity.
| Item | Weight | Value |
|——|——–|——-|
| A | 2 | $10 |
| B | 3 | $15 |
| C | 5 | $20 |
| D | 7 | $30 |
Huffman Coding: Character Frequencies
Huffman coding is a lossless data compression algorithm that assigns variable-length codes to characters based on their frequencies. The following table illustrates the frequencies of characters in a given text, showcasing the importance of assigning shorter codes to frequently occurring characters.
| Character | Frequency |
|———–|———–|
| A | 15% |
| B | 7% |
| C | 12% |
| D | 20% |
| E | 35% |
| F | 11% |
Job Sequencing with Deadlines
In the realm of job sequencing, greedy algorithms play a crucial role in determining the maximum profit. This table demonstrates the deadlines and corresponding profits of various jobs, highlighting the importance of selecting jobs optimally based on their deadlines and profits to maximize the overall gain.
| Job | Deadline | Profit |
|——-|———-|——–|
| A | 2 | $10 |
| B | 1 | $15 |
| C | 2 | $20 |
| D | 1 | $30 |
Activity Selection
When it comes to scheduling activities, a greedy algorithm helps us make optimal choices, maximizing the number of activities that can be performed simultaneously. This table showcases the start and finish times of various activities, demonstrating the strategy of scheduling the activities in a non-decreasing order of finish times.
| Activity | Start Time | Finish Time |
|———-|————|————-|
| A | 10:00 AM | 11:30 AM |
| B | 10:30 AM | 12:00 PM |
| C | 11:00 AM | 12:30 PM |
| D | 11:30 AM | 1:00 PM |
| E | 12:00 PM | 1:30 PM |
Minimum Spanning Tree
For determining the minimum spanning tree, greedy algorithms prove to be efficient. This table displays the weights of edges in a graph, providing insights into the selection of edges with minimum weight while ensuring the tree spans all the vertices.
| Edge | Weight |
|—————|——–|
| AB (Vertex A to Vertex B) | 2 |
| AC (Vertex A to Vertex C) | 4 |
| AD (Vertex A to Vertex D) | 5 |
| BC (Vertex B to Vertex C) | 3 |
| BD (Vertex B to Vertex D) | 6 |
Interval Scheduling
The greedy approach plays a critical role in interval scheduling problems. This table represents various intervals, emphasizing the choice of intervals with the earliest possible finish time to maximize the number of mutually compatible activities.
| Interval | Start Time | Finish Time |
|———-|————|————-|
| A | 10:00 AM | 11:30 AM |
| B | 10:45 AM | 12:00 PM |
| C | 11:00 AM | 12:30 PM |
| D | 11:30 AM | 1:00 PM |
| E | 12:15 PM | 1:30 PM |
Shortest Superstring Problem
In the context of genome sequence assembly, the greedy algorithm serves as a valuable tool for solving the shortest superstring problem. This table displays a set of strings, demonstrating how to select two strings with maximum overlapping length at each step to find the shortest superstring.
| Strings | Overlapping Length |
|—————–|——————–|
| “ABCD” and “BCD” | 3 |
| “CDEF” and “DEF” | 2 |
| “DEFGH” and “GH” | 2 |
| “GHIJ” and “IJ” | 2 |
Fractional Knapsack Problem
The fractional knapsack problem can be efficiently solved using a greedy algorithm. This table showcases the weights, values, and corresponding value-to-weight ratios of items, illustrating the selection of items with the highest value-to-weight ratio until the knapsack is full.
| Item | Weight | Value | Value-to-Weight Ratio |
|——|——–|——-|———————-|
| A | 2 | $10 | $5 |
| B | 3 | $15 | $5 |
| C | 5 | $20 | $4 |
| D | 7 | $30 | $4.28 |
Task Scheduling
In the domain of task scheduling, greedy algorithms help us to minimize the waiting time of processes. This table represents the arrival time and burst time of various tasks, showcasing the importance of scheduling tasks based on their burst time in a non-decreasing order to minimize waiting time.
| Task | Arrival Time | Burst Time |
|——|————–|————|
| A | 1:00 PM | 5 |
| B | 2:00 PM | 4 |
| C | 3:00 PM | 6 |
| D | 4:00 PM | 2 |
In summary, greedy algorithms offer efficient and effective solutions across a range of computational problems. By making locally optimal choices at each step, these algorithms provide viable approximations or even optimal solutions for complex optimization challenges.
Computer Algorithm Greedy
Frequently Asked Questions
- Why is Greedy Algorithm important?
- The Greedy Algorithm is important because it provides an efficient approach to solve optimization problems. It is based on making the locally optimal choice at each step, with the hope that it will lead to a globally optimal solution.
- What is the difference between a Greedy Algorithm and a Dynamic Programming Algorithm?
- A Greedy Algorithm makes the best choice at each step without considering the overall problem, whereas a Dynamic Programming Algorithm breaks down the problem into overlapping subproblems and solves each subproblem only once, storing the solution for future references.
- What are some real-life applications of Greedy Algorithms?
- Greedy Algorithms find applications in various fields such as scheduling, networking, resource allocation, data compression, and more. For example, the Dijkstra’s algorithm for finding the shortest path and the Huffman coding for data compression are both based on Greedy Algorithms.
- Can Greedy Algorithms guarantee an optimal solution for all problems?
- No, Greedy Algorithms do not always guarantee the optimal solution for all problems. While they often provide a near-optimal solution, there are cases where a locally optimal choice might lead to a suboptimal or incorrect overall solution.
- What are some characteristics of problems suitable for Greedy Algorithms?
- Some characteristics of problems suitable for Greedy Algorithms include having optimal substructure (a locally optimal choice leads to a globally optimal solution), the greedy choice property (a globally optimal solution can be obtained by making a locally optimal choice), and no constraints or dependencies between subproblems.
- When should I use a Greedy Algorithm over other algorithms?
- You should consider using a Greedy Algorithm when the problem exhibits the appropriate characteristics and the greedy approach guarantees a satisfactory solution. Additionally, Greedy Algorithms are often easier to implement and require less computational resources compared to other algorithms.
- Are there any drawbacks or limitations of Greedy Algorithms?
- Yes, there are drawbacks and limitations to Greedy Algorithms. While they can be efficient and provide good results, they may not always yield the optimal solution. Greedy Algorithms also rely heavily on the choice of the greedy strategy, which can sometimes lead to incorrect or suboptimal solutions.
- Can a Greedy Algorithm be improved or optimized?
- In some cases, a Greedy Algorithm can be improved or optimized by using additional heuristics or incorporating other algorithms. However, it is crucial to carefully analyze the problem and its characteristics to determine if such improvements are applicable.
- Are there any alternatives to Greedy Algorithms?
- Yes, there are alternative algorithms to Greedy Algorithms depending on the problem and its constraints. Some commonly used alternatives include Divide and Conquer algorithms, Dynamic Programming algorithms, and Backtracking algorithms.
- Where can I learn more about Greedy Algorithms?
- You can find more information about Greedy Algorithms in textbooks on algorithms and data structures, online courses or tutorials on algorithm design, and through practice on coding platforms and algorithmic problem-solving websites like LeetCode or HackerRank.