Write Data to JSON File using Python
Python is a popular programming language known for its simplicity and versatility. One of its key features is the ability to work with different data formats, including JSON (JavaScript Object Notation) files. In this article, we will explore how to write data to a JSON file using Python.
Key Takeaways:
- Python provides built-in libraries and modules to work with JSON files.
- JSON is a lightweight data interchange format that is easy to read and write.
- You can use Python to convert data structures into JSON format and write them to a file.
- Writing data to a JSON file in Python involves a few simple steps.
JSON is a popular choice for storing and exchanging data due to its simplicity and compatibility with different programming languages. It is widely used in web development for sending and receiving data between servers and clients. Python provides built-in libraries such as json to work with JSON files efficiently.
Before writing data to a JSON file, it is important to convert the data into a JSON format. In Python, you can use the json.dumps() function to serialize and convert Python objects to a JSON formatted string. This function takes an object as input and returns a JSON string representation of the object. For example:
import json data = {"name": "John", "age": 30, "city": "New York"} json_data = json.dumps(data) print(json_data)
This code block converts a Python dictionary into a JSON string.
Once you have converted the data into a JSON format, you can write it to a JSON file using Python. The process involves opening a file in write mode, converting the data to a JSON format, and writing it to the file. Here is an example:
import json data = {"name": "John", "age": 30, "city": "New York"} with open("data.json", "w") as file: json.dump(data, file)
This code block writes the JSON data to a file named “data.json” in the current directory.
When writing data to a JSON file using Python, it is important to ensure that the file is properly formatted and structured. Python’s json.dump() function takes care of this by automatically indenting the JSON data for better readability. The indent parameter of the json.dump() function can be set to a desired number of spaces to control the indentation. For example:
import json data = {"name": "John", "age": 30, "city": "New York"} with open("data.json", "w") as file: json.dump(data, file, indent=4)
This code block indents the JSON data with 4 spaces for improved readability.
Data Examples
Product | Price (USD) |
---|---|
Product A | 10.99 |
Product B | 15.49 |
Product C | 25.99 |
Table 1: Example of product data in a JSON format.
- Create a Python dictionary or data structure to represent the data you want to write to a JSON file.
- Convert the data structure into a JSON formatted string using the json.dumps() function.
- Open a file in write mode using open().
- Use the json.dump() function to write the JSON data to the file.
- Close the file using the close() method to save the changes.
Summary
In conclusion, writing data to a JSON file using Python is a straightforward process. Python provides built-in libraries and modules to handle JSON files efficiently. By following a few simple steps, you can convert Python objects into a JSON format and write them to a file. JSON is a versatile data format that is widely used in various applications, making it an essential skill for any Python developer.

Common Misconceptions
Misconception 1: Writing data to a JSON file is a complex task
One common misconception about writing data to a JSON file using Python is that it is a complex task that requires extensive programming knowledge. However, this is not the case. Python provides a simple and easy-to-understand JSON library that allows users to read and write data to JSON files with just a few lines of code.
- Python’s JSON library provides easy-to-use functions for reading and writing JSON data.
- There are ample resources and tutorials available online that can guide users through the process of writing data to a JSON file.
- With basic Python knowledge, anyone can easily write data to a JSON file.
Misconception 2: JSON files can only store simple data types
Another common misconception is that JSON files can only store simple data types such as strings and numbers. While JSON is indeed great for storing simple data, it is also capable of storing more complex data structures. Python’s JSON library allows users to store lists, dictionaries, and even nested data structures in a JSON file.
- JSON files can store complex data structures like lists and dictionaries.
- Nested data structures can be stored in JSON files as well.
- JSON supports a wide range of data types including arrays, objects, strings, numbers, booleans, and null values.
Misconception 3: Writing data to a JSON file requires external libraries
Some people believe that writing data to a JSON file in Python requires the use of external libraries or packages. While there are indeed third-party libraries available for working with JSON, Python’s standard library already includes a built-in JSON module that provides all the necessary functionality for reading and writing JSON files.
- Python’s built-in JSON module provides all the necessary functions for working with JSON files.
- There is no need to install additional libraries or packages for basic JSON file operations.
- The JSON module is part of Python’s standard library, making it readily available to all Python users.
Misconception 4: JSON files are only used for data storage
Many people are under the misconception that JSON files are only useful for storing and transferring data. While JSON is indeed commonly used for these purposes, it has a wide range of applications beyond data storage. JSON files can be used for configuration files, web APIs, and even as a data interchange format between different programming languages.
- JSON files can be used as configuration files for Python applications.
- Web APIs often use JSON as the data format for data exchange.
- JSON files are language-independent and can be easily read and written by different programming languages.
Misconception 5: Writing data to a JSON file requires expert-level programming skills
Some people believe that writing data to a JSON file is a task that can only be accomplished by programmers with expert-level skills. This misconception often discourages beginners from attempting to write data to JSON files. However, as mentioned earlier, Python’s JSON library offers a simple and easy-to-understand interface that allows users of all skill levels to write data to JSON files.
- Python’s JSON library is designed with simplicity in mind, making it accessible to beginners.
- There are plenty of code examples and tutorials available online that can guide beginners through the process of writing data to a JSON file.
- With basic programming knowledge, anyone can successfully write data to a JSON file using Python.
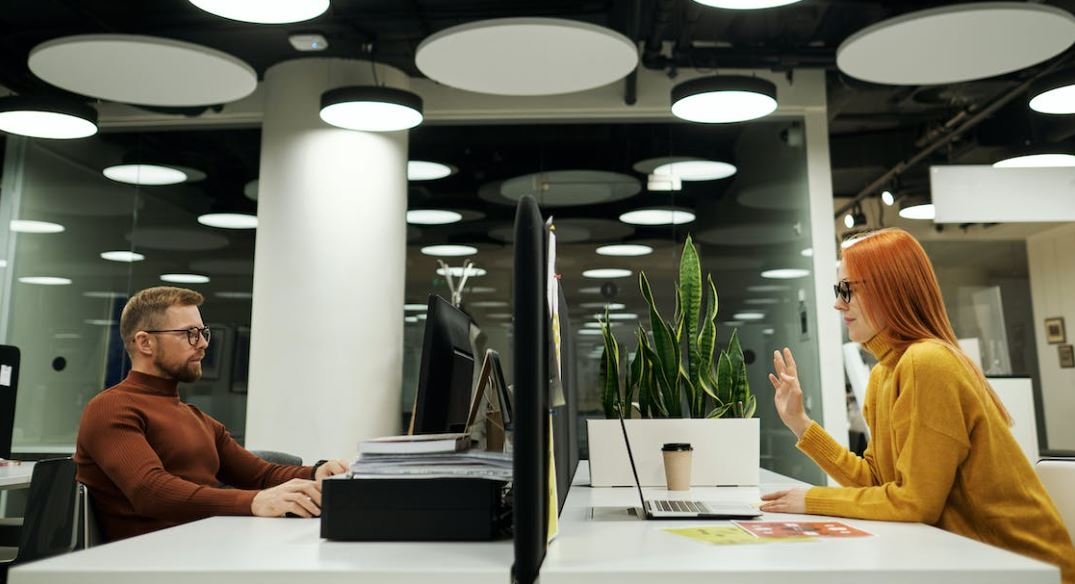
Python Libraries
Python offers a wide range of libraries that provide powerful tools for handling and manipulating data. One such library is the pandas library, which allows us to read, write, and analyze data in various formats, including JSON. In the following tables, we will explore different methods of writing data to a JSON file using Python.
Method 1: Using the JSON module
The JSON module in Python provides a straightforward method for writing data to a JSON file. By importing the module and using the json.dump()
function, we can easily convert Python data into a JSON format and save it.
Method 2: Writing a Python dictionary to JSON
Python dictionaries are versatile data structures that can be easily written to a JSON file. By using the json.dump()
function and passing a dictionary as the data source, we can store the key-value pairs in JSON format for later use.
Method 3: Writing a Python list of dictionaries to JSON
If we have a list that contains multiple dictionaries, we can save this data structure to a JSON file as well. The json.dump()
function allows us to handle complex data structures and convert them into JSON format efficiently.
Method 4: Creating a DataFrame and writing it to JSON
The pandas library provides a high-performance data structure called DataFrame, which is capable of storing and processing large datasets. By creating a DataFrame and using its to_json()
method, we can easily write the contents of the DataFrame to a JSON file.
Method 5: Writing data to a nested JSON structure
In some cases, our data may have a hierarchical or nested structure. Python allows us to handle such scenarios by creating a nested dictionary and writing it to a JSON file. This approach ensures that the resulting JSON file maintains the intended structure of the data.
Method 6: Appending data to an existing JSON file
Instead of overwriting an entire JSON file, we can also append data to it incrementally. By opening the JSON file in append mode and using the json.dump()
function, any new data can be added to the existing JSON file without modifying the existing contents.
Method 7: Indenting JSON output
By default, JSON files are not formatted with indentation, making them less readable. However, Python provides an option to enable indentation while writing data to a JSON file. By specifying the indent
parameter in the json.dump()
function, we can greatly improve the readability of the resulting JSON file.
Method 8: Handling errors during JSON writing
It is essential to properly handle errors that might occur during the process of writing data to a JSON file. By utilizing exception handling with try-except
blocks, we can catch and manage any errors that might arise, ensuring a smooth execution of our code.
Method 9: Writing data to JSON in a compact format
In some cases, we may prefer to generate JSON files with minimal whitespace, reducing the overall file size. Python allows us to achieve this by specifying the separators
parameter in the json.dump()
function, controlling the separation of objects, arrays, and other elements.
Method 10: Writing data to JSON files asynchronously
Asynchronous programming can significantly improve the performance of our code when dealing with I/O operations, such as writing data to a JSON file. By utilizing asynchronous libraries and techniques, we can write data to JSON files concurrently, effectively utilizing system resources and minimizing execution time.
With the various methods demonstrated above, Python provides flexible and efficient ways to write data to JSON files. By harnessing the power of libraries and leveraging the strengths of the language, we can handle a wide range of data manipulation tasks effectively.
Frequently Asked Questions
What is JSON? How is it used in Python?
JSON (JavaScript Object Notation) is a lightweight data interchange format that is commonly used to represent structured data. It is easy for humans to read and write, and it is easy for machines to parse and generate. Python provides the built-in json module to work with JSON effectively.
How do I write data to JSON file using Python?
To write data to a JSON file using Python, you can follow these steps:
- Create a Python dictionary or list containing the data you want to write.
- Import the json module.
- Open a file in write mode using the open() function.
- Use the json.dump() or json.dumps() method to convert the data into a JSON string.
- Write the JSON string to the file.
- Close the file.
Can you provide an example of writing data to a JSON file?
Sure! Here’s an example:
import json data = { "name": "John Doe", "age": 25, "city": "New York" } with open("data.json", "w") as file: json.dump(data, file)
What is the difference between json.dump() and json.dumps() methods?
The json.dump() method directly writes the JSON data to a file-like object, while the json.dumps() method returns a JSON string representation of the data. You can then decide how to handle the JSON string, such as writing it to a file or sending it over a network.
Can I write data to an existing JSON file using Python?
Yes, you can write data to an existing JSON file by opening it in write mode and using the appropriate json.dump() or json.dumps() method to overwrite the existing data or append new data to it.
How do I write formatted/indented JSON data using Python?
To write JSON data in a formatted/indented manner, you can pass the `indent` parameter to the json.dump() or json.dumps() method and specify the number of spaces you want to use for indentation. For example, `json.dump(data, file, indent=4)` will write the JSON data with each level of nesting indented by 4 spaces.
What should I do if I encounter an error while writing data to a JSON file?
If you encounter an error while writing data to a JSON file, you should check the error message and the problematic code section. Common errors can include file permission issues, incorrect JSON syntax, or invalid data types. Reviewing and debugging your code can help identify and resolve the issue.
Can I write non-primitive data types, such as custom objects, to a JSON file?
Yes, you can write non-primitive data types, such as custom objects, to a JSON file. However, the object needs to be serializable, meaning it can be converted to a JSON-supported data type. You can use the json module’s default and custom JSON encoders/decoders or define your own serialization logic to handle non-primitive data types.
Are there any limitations or considerations when writing data to a JSON file in Python?
There are a few limitations and considerations to keep in mind when writing data to a JSON file using Python:
- JSON does not support certain data types like complex numbers or binary data. You may need to handle these types separately.
- Be cautious when handling large datasets, as reading and writing large JSON files can consume substantial memory and processing power.
- Ensure the JSON data complies with the desired schema or structure to avoid unexpected issues when reading or manipulating the data later.
Where can I find more information about writing data to a JSON file using Python?
You can find more information about writing data to a JSON file using Python in the official Python documentation for the json module. Additionally, online tutorials, forums, and books on Python programming can provide further insights and examples.