Output Data to CSV Using Python
Python is a versatile programming language that allows you to perform various tasks, including manipulating data. One common task is outputting data to a CSV (Comma Separated Values) file. This article will guide you through the process of using Python to output data to a CSV file, providing you with the necessary tools to efficiently organize and store your data.
Key Takeaways:
- Python can be used to output data to a CSV file.
- CSV files are useful for organizing and storing data in a tabular format.
- Python’s csv module provides functions for working with CSV files.
- Using the writerow() method, you can write each row of data to the CSV file.
- Always remember to close the CSV file after writing data to it.
When working with data in Python, it is often necessary to convert the data into a more accessible format. One common format used for data storage is the CSV format. CSV files are plain text files that contain data values separated by commas. They are commonly used for importing and exporting data between different programs.
To output data to a CSV file in Python, the first step is to import the csv
module. This module provides functions for reading and writing CSV files. Once imported, you can create a new CSV file using the open()
function with the file mode set to 'w'
or write mode. This will create a new file or overwrite an existing file with the same name.
For example, you can use the following code to create a new CSV file named “data.csv”:
import csv
with open('data.csv', 'w', newline='') as file:
# Perform operations on the file
pass
After creating the CSV file, you can start writing data to it. The csv
module provides a writer
object that allows you to easily write data to the file. You can create a writer object by calling the writer()
function, passing the file object as a parameter. Once created, you can use the writer object’s writerow()
method to write each row of data to the file.
For example, let’s output a list of employee information to a CSV file:
import csv
# Employee data
employees = [
['John Doe', 35, 'john.doe@example.com'],
['Jane Smith', 28, 'jane.smith@example.com'],
['Mike Johnson', 42, 'mike.johnson@example.com'],
]
with open('employees.csv', 'w', newline='') as file:
writer = csv.writer(file)
for employee in employees:
writer.writerow(employee)
Table 1: Data Exported to employees.csv
Name | Age | |
---|---|---|
John Doe | 35 | john.doe@example.com |
Jane Smith | 28 | jane.smith@example.com |
Mike Johnson | 42 | mike.johnson@example.com |
Once you have finished writing data to the CSV file, it is important to close the file to ensure that all data is properly saved. You can do this by calling the close()
method on the file object, or by using the with
statement as shown in the previous examples. This will also free up any system resources allocated to the file.
Remember to close the file after writing data:
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
# Write data to the file
# File is automatically closed outside the 'with' block
By using Python to output data to a CSV file, you can efficiently organize and store your data in a structured manner. CSV files are widely supported and can be easily imported into other programs or systems. Furthermore, Python’s built-in csv
module provides a straightforward and intuitive way to handle CSV files, making it a powerful tool for working with tabular data.
Table 2: Data Exported to sales.csv
Product | Quantity | Price |
---|---|---|
Apple | 10 | 0.99 |
Orange | 5 | 1.50 |
Banana | 8 | 0.50 |
In addition to writing plain data to a CSV file, the csv
module allows you to write more complex data structures, such as dictionaries and lists of objects. By using the writerow()
method along with list comprehensions or loops, you can easily export structured data to a CSV file.
For example, let’s export a list of dictionaries representing sales data:
import csv
# Sales data
sales = [
{'Product': 'Apple', 'Quantity': 10, 'Price': 0.99},
{'Product': 'Orange', 'Quantity': 5, 'Price': 1.50},
{'Product': 'Banana', 'Quantity': 8, 'Price': 0.50},
]
with open('sales.csv', 'w', newline='') as file:
fieldnames = ['Product', 'Quantity', 'Price']
writer = csv.DictWriter(file, fieldnames=fieldnames)
writer.writeheader()
for sale in sales:
writer.writerow(sale)
Table 3: Data Exported to sales.csv
Product | Quantity | Price |
---|---|---|
Apple | 10 | 0.99 |
Orange | 5 | 1.50 |
Banana | 8 | 0.50 |
To summarize, Python offers the csv
module for easily outputting data to a CSV file. You can use the writerow()
method to write each row of data to the file, and remember to close the file when finished. By leveraging Python’s capabilities, you can efficiently organize and store your data in a well-structured format, enabling easy integration with other systems.
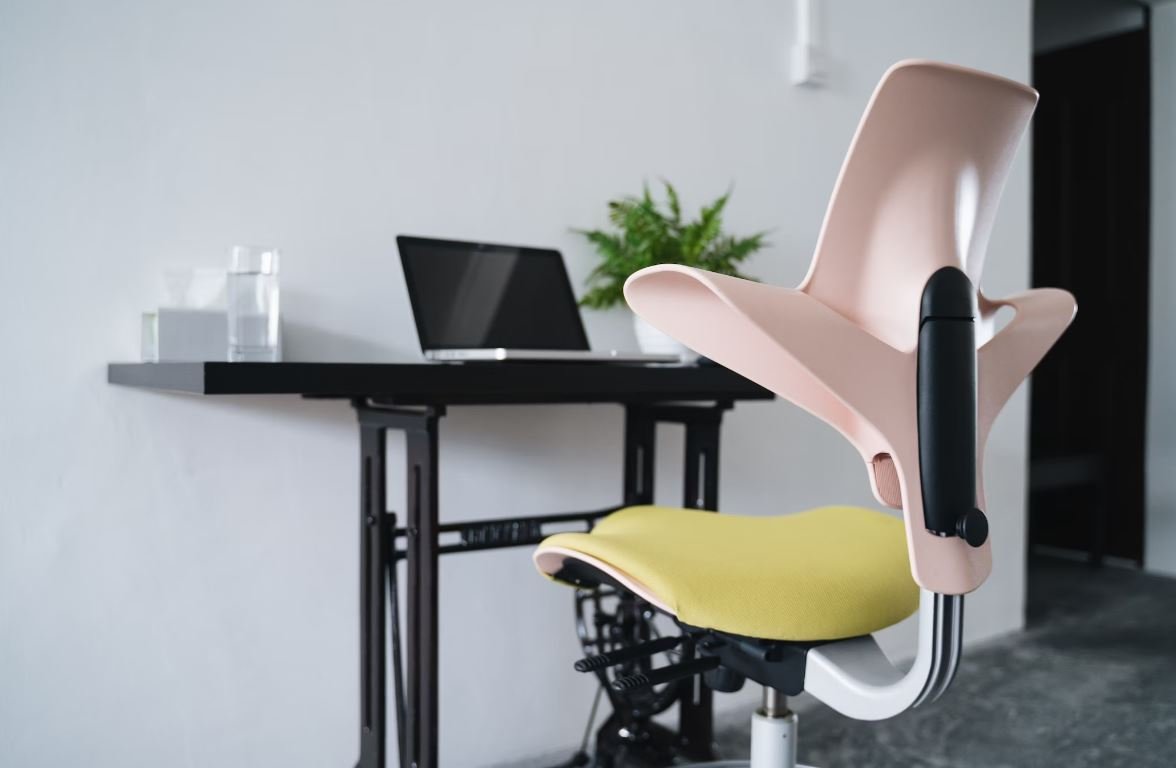
Common Misconceptions
1. CSV is a complex file format
One common misconception is that CSV (Comma Separated Values) is a complex file format that requires specialized tools or programs to work with. However, CSV is actually a very simple and straightforward format that can be easily created and manipulated using just a text editor or a programming language like Python.
- CSV files consist of simple plain-text data separated by commas or other delimiters such as tabs.
- CSV files can be easily created from spreadsheet software like Microsoft Excel or Google Sheets by saving the file in the CSV format.
- Python provides built-in libraries and modules like
csv
that make working with CSV files easy and efficient.
2. CSV files can only store basic data types
Another misconception is that CSV files can only store basic data types such as numbers and text. While it is true that CSV files are commonly used for storing tabular data, they can actually accommodate a wide range of data types and structures.
- CSV files can store numbers, strings, dates, and other basic data types.
- CSV files can also handle more complex data structures like nested lists or dictionaries, as long as the data is properly formatted and separated by delimiters.
- Python’s
csv
module provides various methods for reading and writing different data types in CSV format, including handling special characters and escaping quotes.
3. CSV files are not suitable for large datasets
Some people mistakenly believe that CSV files are not suitable for handling large datasets, assuming that their simplicity and plain-text nature make them inefficient or incapable of handling large amounts of data. However, CSV files can be just as effective as other file formats for handling large datasets.
- CSV files can be compressed to reduce their file size, making them more efficient for storage and transportation.
- CSV files can be easily split into multiple smaller files or processed in chunks to avoid memory limitations when dealing with large datasets.
- Python’s
pandas
library provides powerful tools for efficiently working with large CSV files, including loading and querying data in chunks or using selective loading techniques.
4. CSV files lack data integrity and validation
Another misconception is that CSV files lack data integrity and validation capabilities, as they are simply plain-text files without any built-in structure or constraints. While CSV files do not inherently enforce data integrity like a relational database, it is still possible to enforce data validation and ensure data integrity when working with CSV files in Python.
- Python can validate and clean the data before writing it to a CSV file, ensuring its integrity and consistency.
- Python’s
csv
module supports various techniques for data validation, including type checking, range validation, and pattern matching. - By combining Python’s data validation capabilities with other libraries like
pandas
ornumpy
, additional data cleanliness and integrity checks can be performed.
5. CSV files are not suitable for complex data analysis
Many people believe that CSV files are too basic and limited for complex data analysis tasks, assuming that more advanced file formats like Excel or databases are required. However, CSV files can be effectively used for complex data analysis tasks using Python.
- Python’s extensive data science libraries like
pandas
,numpy
, andmatplotlib
can easily read, manipulate, and analyze data stored in CSV files. - CSV files can be easily imported into data analysis tools like Jupyter Notebook or popular software for statistical analysis.
- With Python, complex data transformations, aggregations, and visualizations can be performed on CSV data without the need for converting to other file formats.
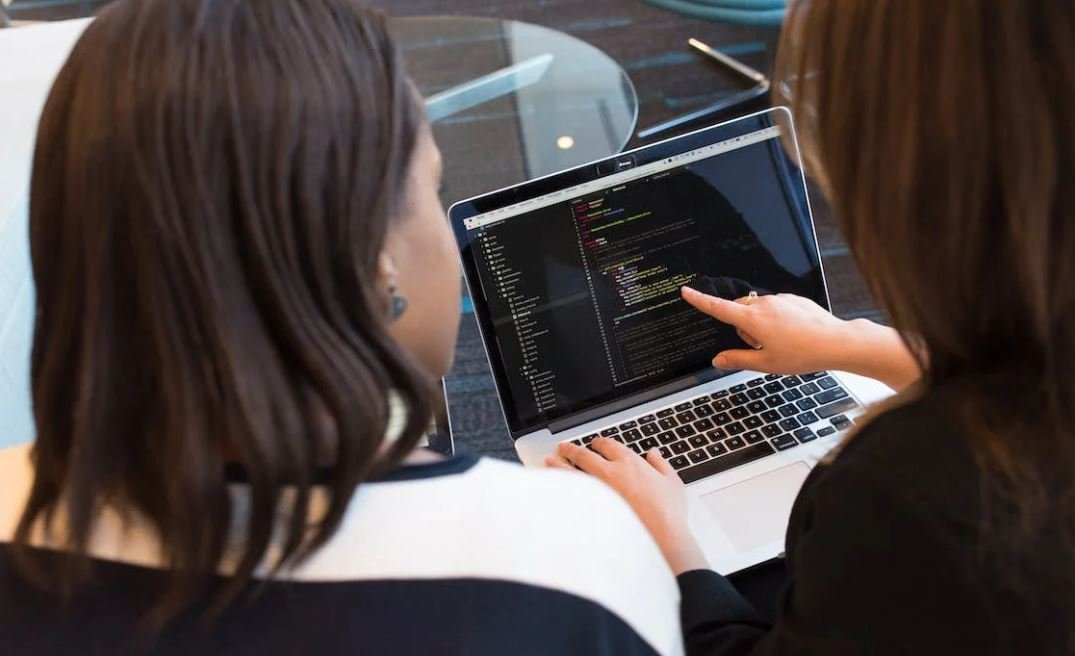
Top 10 Movies Grossing Worldwide
As streaming platforms continue to rise in popularity, the box office numbers for movies have become increasingly important. Here we present the top 10 movies that have grossed the highest worldwide.
Movie | Gross Amount (in billions) |
---|---|
Avengers: Endgame | 2.798 |
Avatar | 2.790 |
Titanic | 2.195 |
Star Wars: The Force Awakens | 2.068 |
Avengers: Infinity War | 2.048 |
Jurassic World | 1.671 |
The Lion King (2019) | 1.663 |
The Avengers | 1.518 |
Furious 7 | 1.516 |
Avengers: Age of Ultron | 1.402 |
Top 5 Highest-Paid Actors in Hollywood
Hollywood is known for its glamorous lifestyles, but it also comes with substantial paychecks. These are the top 5 highest-paid actors in Hollywood.
Actor | Earnings (in millions) |
---|---|
Dwayne Johnson | 89.4 |
Ryan Reynolds | 71.5 |
Mark Wahlberg | 58 |
Ben Affleck | 55 |
Vin Diesel | 54 |
Costliest Tech Acquisitions in History
The tech industry is no stranger to blockbuster deals. These are the costliest tech acquisitions of all time.
Company | Acquiring Company | Deal Amount (in billions) |
---|---|---|
Arm Holdings | SoftBank Group | 31.4 |
Guthy-Renker | Procter & Gamble | 30.0 |
Red Hat | IBM | 34.0 |
Microsoft | 26.2 | |
Motorola Mobility | 12.5 |
Global Internet Users by Region
The internet has bridged the gaps between countries and continents. Here is the breakdown of global internet users by region.
Region | Internet Users (in millions) |
---|---|
Asia | 2,549.9 |
Europe | 727.3 |
Africa | 553.1 |
Americas | 414.4 |
Oceania/Australia | 290.6 |
Top 5 Richest Billionaires
Fortunes are made and lost in the world of business. Here are the top 5 richest billionaires in the world.
Billionaire | Wealth (in billions) |
---|---|
Jeff Bezos | 193.4 |
Elon Musk | 184.3 |
Bernard Arnault & Family | 181.6 |
Bill Gates | 150.1 |
Mark Zuckerberg | 138.8 |
World’s Tallest Buildings
Architectural marvels reach for the sky, showcasing human ingenuity. These are the world’s tallest buildings.
Building | Height (in meters) |
---|---|
Burj Khalifa | 828 |
Shanghai Tower | 632 |
Abraj Al-Bait Clock Tower | 601 |
Ping An Finance Center | 599 |
Lotte World Tower | 555 |
Most Popular Social Media Platforms
Social media platforms have transformed the way we connect and share information. These are the most popular social media platforms based on active users.
Platform | Active Users (in millions) |
---|---|
2,853 | |
YouTube | 2,291 |
2,000 | |
Messenger (Facebook) | 1,300 |
1,206 |
Top 5 Largest E-commerce Companies
The convenience of online shopping has made e-commerce one of the most rapidly growing industries. These are the top 5 largest e-commerce companies.
Company | Market Value (in billions) |
---|---|
Amazon | 1,839.6 |
Alibaba | 609.8 |
Tencent | 599.2 |
Shopify | 159.0 |
JD.com | 122.6 |
Countries with the Highest Life Expectancy
Access to quality healthcare and advancements in medicine contribute to the life expectancy of a nation. These countries have the highest life expectancy.
Country | Life Expectancy (in years) |
---|---|
Japan | 83.7 |
Switzerland | 83.4 |
Spain | 83.1 |
Australia | 82.8 |
Italy | 82.7 |
From the highest-grossing movies to the longest-living nations, data reveals fascinating insights. Whether it’s the immense wealth of billionaires or the growth of e-commerce, these tables highlight significant aspects of our world. The numbers offer a glimpse into the trends and successes that shape our society. As technology evolves and access to information becomes more prevalent, the importance of accurate data analysis grows. By examining these visual representations of information, we gain a deeper understanding of the world around us.
Frequently Asked Questions
How can I output data to CSV using Python?
What is CSV?
What are the benefits of using Python to output data to CSV?
Can Python handle large datasets?
How do I install the required packages for outputting data to CSV in Python?
What is the command to install pandas?
What is the basic process for outputting data to CSV using Python?
What are the steps to write data to a CSV file?
2. Create a CSV writer object using the ‘csv.writer()’ function.
3. Write the desired data to the CSV file using the writer object’s ‘writerow()’ method.
4. Close the CSV file using the file object’s ‘close()’ method.
How can I read data from a CSV file in Python?
What is the command to read a CSV file using pandas?
Can I customize the delimiter used in the CSV file?
How can I change the delimiter used in the CSV file?
What if my data contains special characters or newlines?
How to handle special characters or newlines in data while outputting to CSV?
Can I append data to an existing CSV file?
Is it possible to append data to an existing CSV file using Python?
What are some common errors encountered when outputting data to CSV using Python?
Why am I getting a ‘Permission Denied’ error while writing to a CSV file?
Are there any alternative formats to CSV for storing tabular data?
What are some alternative formats to CSV?