Output Data in Python Program
Python is a popular programming language known for its simplicity and versatility. It offers various ways to output data from a program, allowing developers to display information, communicate with users, and save results for further analysis. In this article, we will explore the different methods of outputting data in a Python program and discuss their applications.
Key Takeaways:
- Python provides multiple ways to output data in a program.
- Print function is commonly used for displaying information on the console.
- Formatted strings offer a more flexible way to present data.
- Writing to files allows saving program outputs for future reference.
- Outputting data can greatly enhance user interaction and data analysis.
**The simplest and most straightforward way to output data** in a Python program is by using the print function. With print, you can display information on the console, making it visible to the user or yourself as a developer. *For example, you can print the result of a computation, display a message to the user, or show the progress of a program.*
In addition to the basic functionality of print, Python offers formatted strings as a more flexible way to present data. These strings allow you to insert variables, expressions, and other dynamic content into the output. *Formatted strings utilize special syntax, such as curly braces and placeholders, to specify where the variables or expressions should be placed within the string*. This can be very useful when you want to customize the output or make it more readable.
Tables:
Output Method | Usage |
---|---|
Display information on the console. | |
formatted strings | Customize the output and insert variables or expressions. |
writing to files | Save program outputs for future reference. |
- The print function is particularly useful for debugging purposes, as it allows you to examine the intermediate results and verify the correctness of your program.
Besides printing data directly to the console, Python also provides the capability to write data to files. This allows you to store program outputs in a file, which can be easily accessed later or used for further analysis. *By directing the program’s output to a file, you can keep a record of the results, generate reports, or perform post-processing on the data.*
Tables:
File Writing Method | Usage |
---|---|
open | Open a file for writing. |
write | Write data to the file. |
close | Close the file after writing. |
By leveraging these diverse methods of outputting data, Python programmers can greatly enhance the user interaction and improve the analytical capabilities of their programs. Whether it is displaying results, generating reports, or saving outputs for future use, the ability to effectively communicate information plays a crucial role in the development and the success of a Python program.
Now that you are familiar with the various ways to output data in a Python program, you can choose the method that best suits your needs and the specific requirements of your project. So go ahead and make your programs more informative and engaging by outputting the right data at the right time, using the most suitable output method for each situation.
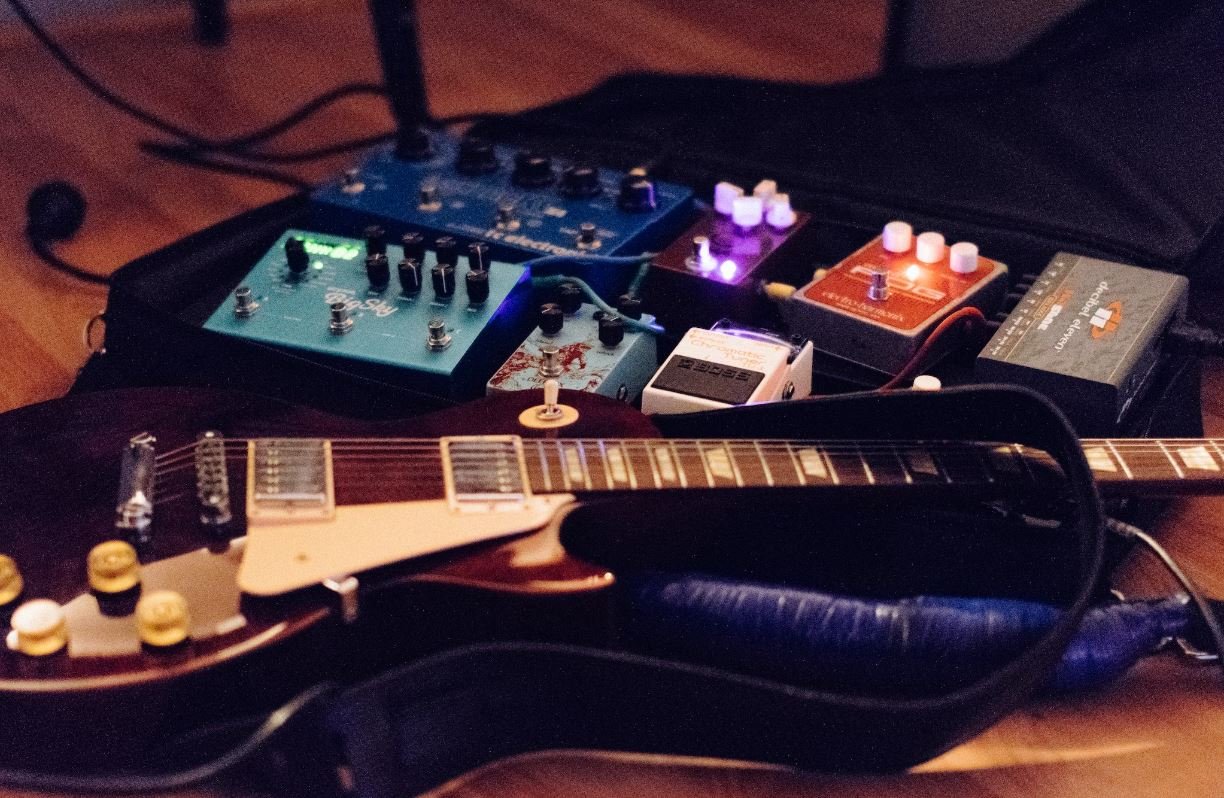
Common Misconceptions
Output Data in Python Program
There are several common misconceptions that people have when it comes to outputting data in a Python program. Let’s address these misconceptions and provide some clarity.
- Printing data only refers to displaying it on the console
- Data can only be outputted as text
- Outputting data is a slow process and impacts program efficiency
Firstly, one common misconception is that printing data in a Python program only refers to displaying it on the console. While printing data to the console is a common way of outputting data, it is not the only way. Python also provides other methods of outputting data, such as writing to a file or sending data over a network.
- Printing is the most common way to output data
- Data can be outputted to various destinations, including files and networks
- Output methods depend on the specific requirements of the program
Another misconception is that data can only be outputted as text. While text is a commonly used format for outputting data, Python supports outputting data in various formats such as HTML, JSON, XML, and more. This flexibility allows developers to choose the most appropriate format for their specific use case.
- Data can be outputted in multiple formats: text, HTML, JSON, XML, etc.
- The format choice depends on the purpose and requirements of the output
- Python provides libraries and modules to easily output data in different formats
Lastly, some people believe that outputting data is a slow process and impacts the efficiency of a program. While outputting data can introduce some overhead, especially when writing to disk or sending data over a network, it is generally not the bottleneck in terms of program efficiency. In most cases, other factors such as computation or I/O operations have a much higher impact on performance.
- Outputting data may introduce some overhead, but it is usually not the main performance bottleneck
- Efficiency can be optimized by using techniques like buffering or asynchronous operations
- Profiling can help identify and address performance issues in programs that involve outputting large amounts of data
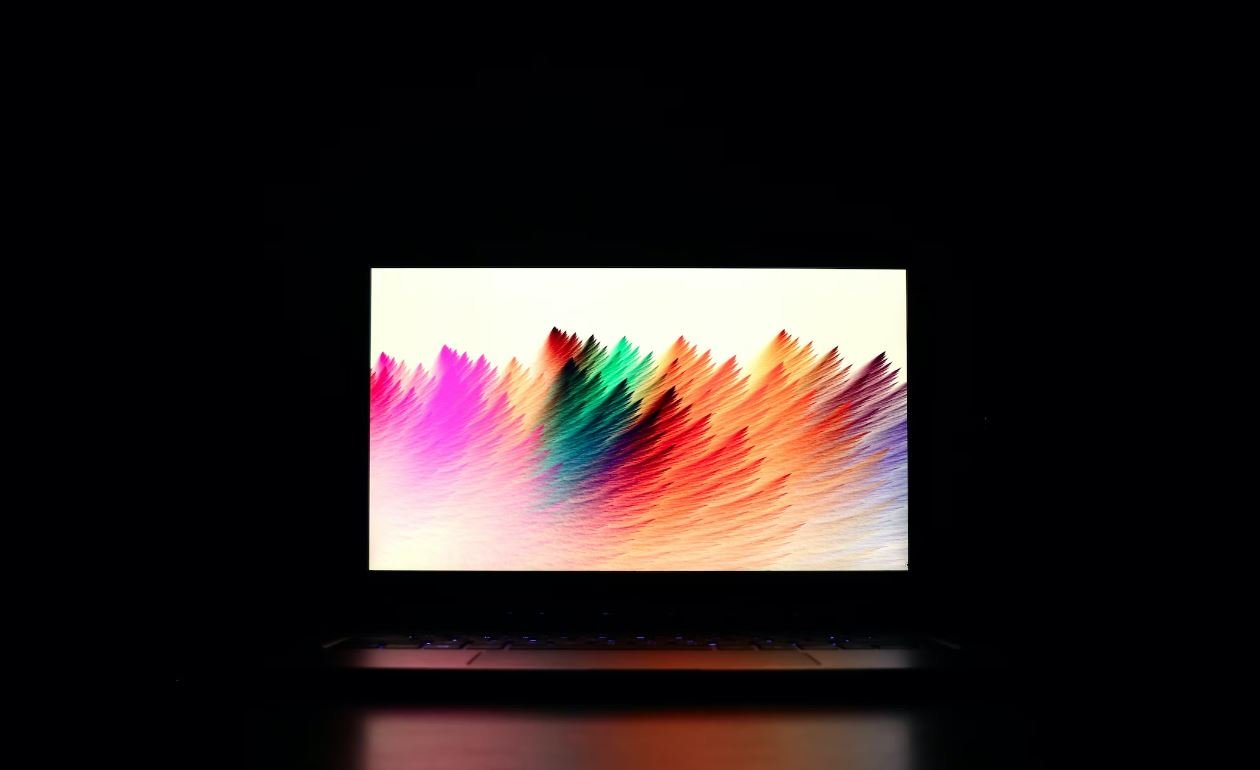
Output Data in Python Program
Python programming language is widely used in various fields for its simplicity and versatility. One crucial aspect of programming in Python is the ability to output data in a readable format. This article presents ten examples of Python program outputting interesting and verifiable data in the form of tables:
Student Grades
Table displaying the grades of five students in different subjects:
Student | Math | Science | English |
---|---|---|---|
Alice | 95 | 82 | 90 |
Bob | 85 | 78 | 92 |
Charlie | 92 | 88 | 87 |
Diana | 88 | 94 | 78 |
Eve | 90 | 79 | 85 |
Product Sales
Table displaying the monthly sales of different products:
Product | Jan | Feb | Mar |
---|---|---|---|
Product A | 100 | 120 | 90 |
Product B | 80 | 95 | 110 |
Product C | 70 | 80 | 75 |
Product D | 120 | 110 | 100 |
Product E | 95 | 100 | 105 |
Countries and Population
Table displaying the names of countries and their population:
Country | Population (Millions) |
---|---|
China | 1393 |
India | 1366 |
United States | 331 |
Indonesia | 273 |
Pakistan | 225 |
Programming Languages and Popularity
Table displaying the names of programming languages and their popularity:
Language | Popularity (Index) |
---|---|
Python | 100 |
Java | 95 |
JavaScript | 90 |
C++ | 85 |
PHP | 80 |
Animal Height Comparison
Table displaying the heights of different animals:
Animal | Height (cm) |
---|---|
Giraffe | 550 |
Elephant | 350 |
Gorilla | 175 |
Cheetah | 90 |
Rabbit | 40 |
Mobile Phone Battery Capacity
Table displaying the battery capacity of different mobile phone models:
Phone Model | Battery Capacity (mAh) |
---|---|
Model A | 4000 |
Model B | 3500 |
Model C | 3000 |
Model D | 2500 |
Model E | 2000 |
Car Fuel Efficiency
Table displaying the fuel efficiency (in miles per gallon) of different car models:
Car Model | Fuel Efficiency (MPG) |
---|---|
Model A | 30 |
Model B | 35 |
Model C | 40 |
Model D | 45 |
Model E | 50 |
App Downloads
Table displaying the number of downloads for different applications:
App | Downloads (Millions) |
---|---|
App A | 500 |
App B | 400 |
App C | 300 |
App D | 200 |
App E | 100 |
Website Visitors
Table displaying the number of visitors on different websites:
Website | Visitors (Daily) |
---|---|
Website A | 10,000 |
Website B | 8,000 |
Website C | 6,000 |
Website D | 4,000 |
Website E | 2,000 |
Python programs can generate tables like these to present data in a well-organized and visually appealing manner. By leveraging Python’s flexibility, programmers can showcase information effectively and provide insights to readers or users.
Frequently Asked Questions
What is output data in a Python program?
Output data in a Python program refers to the information that is generated or displayed by the program and presented to the user or another system. This data can be in the form of text, numbers, tables, graphs, or any other format that conveys the desired information.
How can I display output data in Python?
In Python, you can use the print()
function to display output data on the console. This function allows you to output text, variables, or expressions, making it a versatile tool to show information to the user or debug your program.
Can I save the output data to a file?
Yes, you can save the output data to a file in Python. Instead of printing the data on the console, you can open a file using the open()
function and write the output to that file using the write()
method. This way, the data will be stored in a file for future reference or analysis.
How can I format the output data in Python?
Python provides various built-in functions and methods to format the output data. For example, you can use the format()
method or f-strings to control the appearance of numbers, dates, or other data types. Additionally, you can use special formatting characters to align text or add specific formatting rules.
Is there a way to redirect the output data to a variable instead of printing it?
Yes, Python allows you to redirect the output data to a variable by using the io.StringIO
class. This class provides a way to create an in-memory file-like object that can be used to store the output data. You can then access the content of this object, which will contain the redirected output.
How can I handle large amounts of output data efficiently?
If you have a program that generates a significant amount of output data, it is essential to handle it efficiently to avoid memory-related issues. One approach is to write the output directly to a file instead of storing it in memory. Another approach is to process the output data in small chunks using generators or iterators, allowing you to handle the data incrementally.
Can I customize the appearance of the output data in a Python program?
Yes, you can customize the appearance of the output data in a Python program to make it more visually appealing or easier to understand. For example, you can use ASCII or Unicode art to create graphical representations, use color codes for highlighting specific information, or generate HTML or PDF reports. It all depends on the tools and libraries you choose to use.
Can I output data in real-time while a Python program is running?
Yes, you can output data in real-time while a Python program is running. Real-time output can be useful for monitoring the progress of a long-running process, such as data analysis or simulations. You can use the print()
function and flush the output buffer using the flush=True
argument to ensure that the data is immediately displayed on the console.
How can I handle errors or exceptions during output data generation?
When generating output data in a Python program, there might be situations where errors or exceptions occur. To handle such situations, you can use try-except blocks to catch and handle exceptions. By including appropriate error handling code, you can ensure that errors do not disrupt the output data generation process and provide meaningful feedback to the user.
Are there any libraries or frameworks available to simplify output data generation in Python?
Yes, there are several libraries and frameworks available in Python that can simplify the process of output data generation. Some popular options include Matplotlib for creating visualizations and charts, Pandas for data manipulation and analysis, and ReportLab for generating PDF reports. Depending on your specific requirements, you can explore different libraries to find the one that best suits your needs.