Input Data Validation in Java
When working with user inputs, it is crucial to validate the data to ensure its correctness, integrity, and security. In Java, input data validation plays a vital role in preventing errors and vulnerabilities in your applications.
Key Takeaways
- Input data validation is essential for ensuring data correctness and security in Java applications.
- Java provides various methods and libraries for input data validation, such as regular expressions and built-in functions.
- Proper input validation helps prevent common vulnerabilities like SQL injection and cross-site scripting.
**In Java**, there are multiple approaches to input data validation, depending on the specific requirements of your application. The most common validation techniques include **regular expressions** and utilizing built-in **Java libraries**.
**Regular expressions** are powerful tools that allow you to define patterns for validating input data. They are especially useful when dealing with complex data formats, such as email addresses, phone numbers, or URLs. For example, you can use a regular expression to ensure that an email address entered by a user follows the correct formatting.
*By utilizing regular expressions, you can create flexible and robust validation logic, validating complex input patterns with ease.*
Another popular way to validate input data in Java is by using built-in libraries and functions. **The `Scanner` class**, for instance, offers methods like `nextInt()`, `nextDouble()`, and `nextLine()` to read user inputs and validate them based on their expected data types. When a user enters invalid input, these functions provide mechanisms to handle the error gracefully rather than crashing the program.
Validation Methods in Java
Validation Method | Description |
---|---|
Regular Expressions | Allows you to define patterns for validating complex input formats. |
Scanner Class | Provides built-in functions to validate input data based on expected data types. |
**Common validation scenarios** include checking if a given input is a number, validating string lengths, or ensuring the input doesn’t contain harmful characters. By implementing appropriate validation methods in your Java application, you can enhance its reliability and security.
*Validating user inputs is particularly essential when handling sensitive data, such as passwords or financial information, to protect against potential security vulnerabilities.*
In addition to validating input data, **proper error handling** is crucial to provide meaningful feedback to users in case of invalid inputs. This helps users understand the issue and correct their input accordingly. Implementing clear error messages or utilizing exception handling mechanisms can greatly improve the user experience.
Error Handling Strategies
- Display clear and descriptive error messages to guide users in correcting their input.
- Utilize exception handling mechanisms, such as try-catch blocks, to catch and handle validation errors.
- Implement input validation at both the client-side and server-side for enhanced security.
Error Handling Technique | Description |
---|---|
Clear Error Messages | Display descriptive messages to guide users in rectifying their input. |
Exception Handling | Utilize try-catch blocks to catch and handle input validation errors. |
In conclusion, input data validation is a critical step when developing Java applications. By properly validating user inputs, you can ensure the correctness, integrity, and security of your data while preventing common vulnerabilities. Whether utilizing regular expressions, built-in Java libraries, or proper error handling strategies, make input data validation a priority in your development process to build reliable and secure software.

Common Misconceptions
Input Data Validation in Java
There are several common misconceptions people have about input data validation in the Java programming language. Let’s explore them:
- Input data validation is only useful for web applications
- Data validation is a replacement for error handling
- All input data needs to be validated before usage
Validation can only occur on the front end
Another common misconception is that input data validation can only be done on the front end (client-side) of a web application. While client-side validation is important for providing a responsive and user-friendly experience, it should not be relied upon as the sole form of validation. Server-side validation is crucial to ensure data integrity and security.
- Front end validation can be bypassed
- Server-side validation acts as an additional layer of security
- Validation on the back end provides a consistent experience across different clients
Validation slows down the application
A misconception often held is that input data validation slows down the application’s performance. While it is true that validation adds some overhead, the impact is typically negligible. Properly implemented validation can improve the overall reliability and maintainability of the codebase.
- Validation prevents unexpected errors and crashes
- Well-designed validation functions can be efficient
- The benefits of validation outweigh the minimal performance impact
Validation is enough to prevent all security vulnerabilities
Some people mistakenly believe that validating input data is sufficient to prevent all security vulnerabilities in Java applications. While validation is an essential step, it is not a complete solution. Additional security measures, such as input sanitation, output encoding, and implementing secure coding practices, are necessary to mitigate potential risks.
- Validation alone cannot protect against all possible attack vectors
- Input sanitization is required to prevent data contamination
- Secure coding practices must be implemented alongside validation
Validation guarantees data correctness
Validation helps ensure that input data meets specified criteria, but it does not guarantee absolute data correctness. There will always be scenarios where valid data may still produce unexpected results. Ensuring data correctness requires a combination of validation, appropriate business logic, and rigorous testing.
- Valid data doesn’t necessarily imply the desired outcome
- Validation can catch obvious mistakes, but not all edge cases
- Continual testing and refining of validation rules is necessary
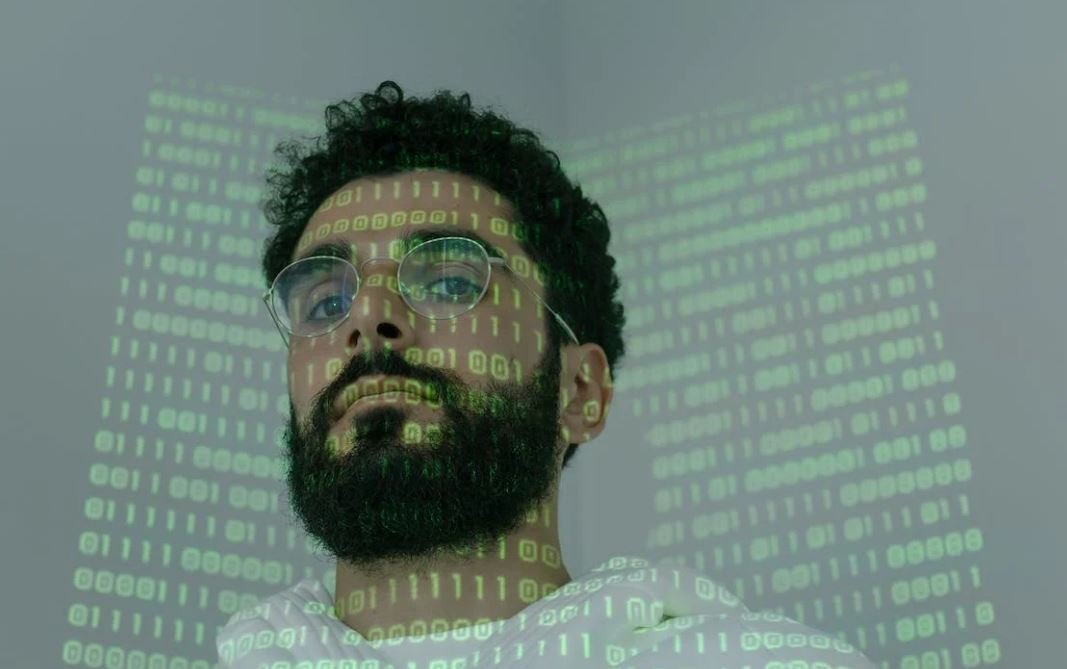
Introduction
Input data validation is an essential aspect of Java programming, ensuring that the data entered by users meets the required criteria. This article explores different validation techniques and concepts in Java, examining their importance in maintaining data integrity and improving overall code reliability.
Table: Primitive Data Types and Valid Ranges
In Java, the primitive data types have specific valid ranges, which must be considered when validating input data.
Table: Regular Expression Patterns
Regular expressions provide a powerful method for validating various types of input data, such as phone numbers, email addresses, and social security numbers.
Table: Input Data Validation Methods
Java offers numerous methods for input data validation, including built-in functions and custom logic.
Table: Web Form Field Validation
Validating user input in web forms is crucial to prevent security vulnerabilities and maintain data consistency.
Table: Error Messages for Invalid Data
Displaying appropriate error messages when invalid data is entered helps users understand and correct their mistakes.
Table: Input Validation Libraries/Frameworks
Several libraries and frameworks are available in Java that simplify input data validation, offering pre-defined validation rules and error handling mechanisms.
Table: Cross-Site Scripting (XSS) Validation
Cross-Site Scripting attacks can be prevented by validating and sanitizing user input, ensuring that no harmful scripts are executed.
Table: Database Input Validation
Validating input data before storing it in a database is crucial to maintain data integrity and prevent SQL injection attacks.
Table: File Input Validation
When handling file input, validating the file type, size, and content ensures the system’s security and stability.
Table: Positive vs. Negative Validation
Considerations on the approaches of positive and negative data validation and their advantages in different scenarios.
Conclusion
Input data validation in Java plays a significant role in ensuring the reliability, security, and integrity of software systems. Through various techniques like regular expressions, error messages, and utilizing libraries or frameworks, developers can enforce strict validation rules, preventing erroneous or malicious data from compromising the application. Accurate input validation contributes to better user experiences, reduces data-related issues, and strengthens the overall stability of Java applications.
Frequently Asked Questions
Input Data Validation in Java