Input Data for Python
Python is a popular programming language that is widely used in various domains, from web development to scientific computing. One of the key aspects of Python programming is working with input data. In this article, we will explore the different ways to input data into Python and how to manipulate and analyze that data.
Key Takeaways:
- Input data is crucial for any data-driven task in Python.
- There are multiple ways to input data into Python, including user input, file input, and database input.
- Data manipulation techniques in Python enable efficient processing and analysis of input data.
In Python, there are several ways to input data into a program. The most basic method is to prompt the user for input using the input()
function. This function allows you to display a message to the user and wait for their input. For example:
“`
name = input(“Enter your name: “)
age = int(input(“Enter your age: “))
“`
*The input()
function can accept any type of input, but it is important to convert it to the appropriate data type if necessary.*
Another common way to input data into Python is through file input. Python provides various ways to read data from files, such as the open()
function and the csv
module. These methods allow you to read data from text files, CSV files, or other file formats. You can then process and analyze the data using Python’s powerful data manipulation libraries such as Pandas.
Tabular Data Examples:
Product | Price | Quantity |
---|---|---|
Apple | 1.99 | 10 |
Orange | 0.99 | 5 |
*Tabular data can be easily imported into Python using libraries like Pandas, which provide convenient functions to read data from different file formats.*
In addition to user input and file input, Python can also read data from databases. Python has modules like sqlite3
and pyodbc
that allow you to connect to databases, execute queries, and retrieve data. With the help of SQL queries, you can extract specific data from large databases and use it for further analysis or processing.
*Python’s database connection capabilities provide seamless integration with existing data sources and enable efficient data handling.*
Numerical Data Example:
Year | Revenue ($) | Profit ($) |
---|---|---|
2018 | 100000 | 20000 |
2019 | 120000 | 25000 |
2020 | 150000 | 30000 |
*Numerical data can be easily visualized and analyzed using Python libraries such as Matplotlib and NumPy.*
Once you have successfully inputted the data, Python offers numerous tools and libraries for data manipulation. For example, you can use the split()
function to split a string into a list of substrings based on a specified delimiter. The join()
function can be used to concatenate a list of strings into a single string. These functions, along with various other string manipulation methods, enable efficient data cleansing and transformation.
*Data manipulation in Python can significantly simplify the process of cleaning, transforming, and preparing data for further analysis.*
Furthermore, Python provides powerful libraries like Pandas and NumPy, which offer extensive data manipulation and analysis capabilities. These libraries enable you to perform advanced operations on input data, such as filtering, sorting, aggregating, and statistical analysis, to gain valuable insights from the data.
Key Python Data Manipulation Libraries:
- Pandas
- NumPy
- Matplotlib
*Python’s data manipulation libraries provide essential tools for performing complex data analysis tasks.*
In conclusion, Python offers various methods to input data, including user input, file input, and database input. Once the data is imported, Python’s robust data manipulation capabilities, supported by libraries like Pandas, allow for efficient processing and analysis. Whether you are working with tabular data or numerical data, Python provides the necessary tools and libraries to handle and analyze your input data effectively.
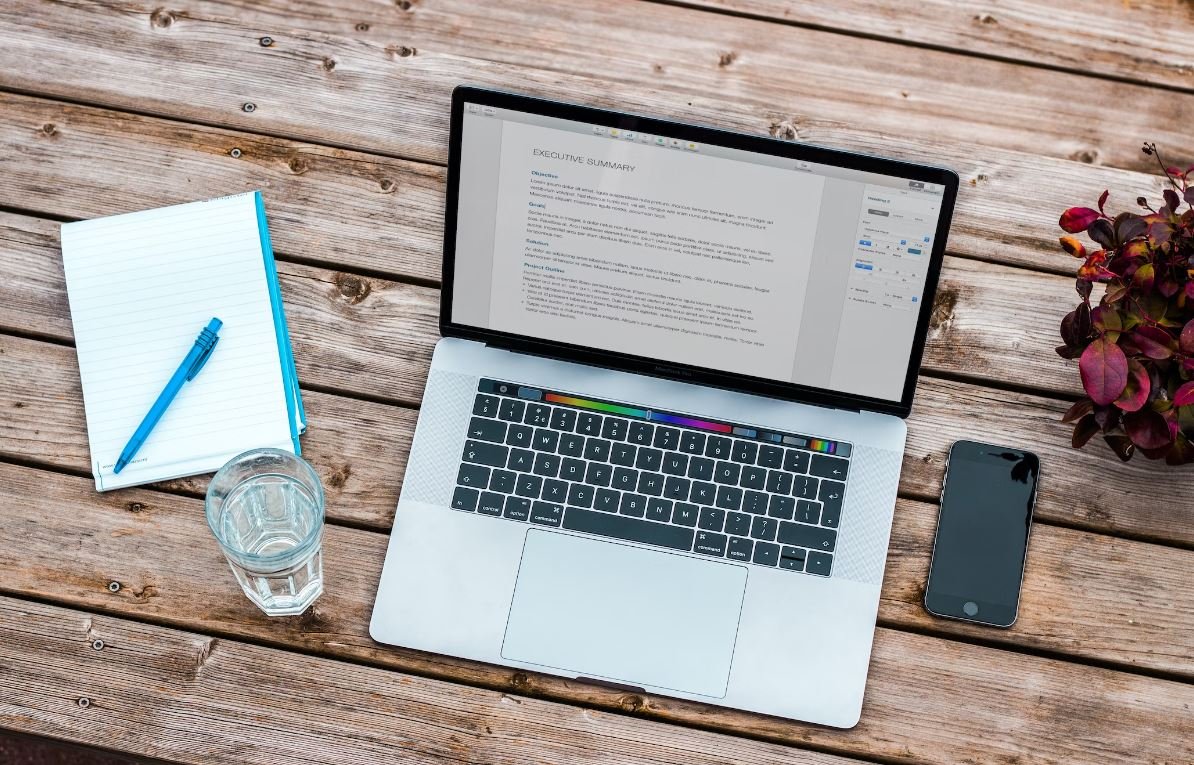
Common Misconceptions
Misconception 1: Input data for Python is always received through user prompts
One common misconception about input data for Python is that it can only be received through user prompts. While user prompts are a common method, there are other ways to input data into a Python program as well.
- Data can be read from a file using Python’s “open” and “read” functions.
- Data can be obtained from an API or web service using Python’s “requests” library.
- Data can be received through a network connection using Python’s socket programming capabilities.
Misconception 2: The input() function always returns a string
Another misconception is that the input() function always returns a string. While it is true that input() returns a string by default, it can be converted to other data types if needed.
- Using the int() function, the string input can be converted to an integer.
- Similarly, the float() function can be used to convert the string input to a floating-point number.
- If the input needs to be treated as a boolean value, the str() or int() function can be used along with appropriate conditional statements.
Misconception 3: Input data must always be provided during runtime
Some people believe that input data for a Python program must always be provided during runtime. However, this is not true as input data can be read from various sources and at different stages of program execution.
- Data can be retrieved from a database before the program is executed.
- Data can be read from a file when the program starts.
- Data can even be received during runtime through user input, API calls, or network connections.
Misconception 4: Input data is always formatted as text
Another misconception is that input data for Python is always expected to be in the form of text. This is not always the case as Python supports other data formats as well.
- Data can be formatted as JSON, and Python’s “json” library can be used to parse the input into Python objects.
- Data can be provided as binary files, which can be read and processed using appropriate Python modules.
- Data can also be received in specific data structures such as lists or dictionaries, which can be directly used in Python programs.
Misconception 5: Input data is always taken from the command line
While many beginners associate input data with command line arguments, it is essential to understand that there are other ways to input data into a Python program.
- Command line arguments can be used, but they are not the only method.
- Data can be passed as program arguments to function calls.
- Data can be obtained interactively through GUI components in Python frameworks such as Tkinter.

Comparing Programming Languages
As developers, we have multiple options when it comes to choosing a programming language for our projects. Each language has its own strengths and weaknesses, making it essential to evaluate their features before making a decision. In this article, we will compare and contrast several popular programming languages based on their syntax, performance, and community support.
Python
Python is a versatile, high-level programming language known for its readability and simplicity. It is often favored by beginners due to its easy-to-understand syntax. With a rich collection of libraries and modules, Python enables developers to tackle a wide range of applications, including web development, data analysis, and artificial intelligence.
Feature | Description |
---|---|
Readability | Python’s elegant and readable code makes it easy to understand and maintain. |
Performance | Although Python offers great productivity, it may not be as fast as some compiled languages. |
Community | The Python community is one of the largest and most active, providing ample support and resources. |
JavaScript
JavaScript is a versatile scripting language primarily used for client-side web development. Its ability to manipulate elements on web pages in real-time makes it a fundamental part of modern web development. With frameworks like React and Angular, JavaScript has expanded its reach to building complex web applications and mobile apps.
Feature | Description |
---|---|
Flexibility | JavaScript’s dynamic nature allows developers to write code that adapts to changing requirements. |
Performance | Modern JavaScript engines have significantly improved runtime performance. |
Community | JavaScript has a vast community that contributes to various open-source projects and provides extensive documentation. |
Java
Java is a powerful, general-purpose programming language known for its platform independence. Java applications can run on any machine or operating system with Java Virtual Machine (JVM) support. It is widely used in enterprise-level software development and Android app development.
Feature | Description |
---|---|
Portability | Java’s “write once, run anywhere” principle allows code to be executed on different platforms without recompiling. |
Performance | Java offers excellent performance due to its ability to optimize bytecode during runtime. |
Community | Java’s vast community provides extensive support and contributes to the development of numerous frameworks and libraries. |
C++
C++ is a powerful, object-oriented programming language widely used for high-performance applications and systems programming. It offers fine-grained control over hardware resources and is a popular choice for tasks that require low-level operations or real-time performance.
Feature | Description |
---|---|
Efficiency | C++ allows developers to write efficient code by directly managing memory and hardware resources. |
Performance | Due to its lightweight nature, C++ code can execute faster than programs written in higher-level languages. |
Community | While not as large as some other communities, the C++ community consists of dedicated experts who provide thorough support. |
Swift
Swift is a modern, statically-typed programming language developed by Apple for iOS, macOS, watchOS, and tvOS app development. It was designed to address the shortcomings of Objective-C and offers a more concise syntax and enhanced safety features.
Feature | Description |
---|---|
Readability | Swift’s syntax is concise and expressive, enabling developers to write clean and maintainable code. |
Performance | Swift is highly efficient and comparable to Objective-C, ensuring fast application execution. |
Community | The Swift community is growing rapidly and provides extensive resources for beginners and experienced developers. |
PHP
PHP is a widely-used server-side scripting language specifically designed for web development. It is embedded within HTML code and can generate dynamic web content and interact with databases. PHP’s simplicity and broad adoption make it a popular choice for building websites and web applications.
Feature | Description |
---|---|
Simplicity | PHP’s intuitive syntax allows beginners to quickly grasp its concepts and start building web applications. |
Performance | Although PHP’s performance has improved over the years, it may not be as fast as other server-side scripting languages. |
Community | PHP has a vast and active community that contributes to frameworks, CMSs, and online forums. |
Rust
Rust is a systems programming language created by Mozilla that focuses on safety, speed, and concurrency. It is designed to prevent common programming errors such as null pointer references and data races. Rust’s strict guarantees make it suitable for low-level programming and building performance-critical software.
Feature | Description |
---|---|
Memory Safety | Rust’s ownership model ensures memory safety without garbage collection or manual memory management. |
Performance | Rust compiles to highly optimized native code, offering comparable performance to C and C++. |
Community | Rust has a passionate and welcoming community that actively contributes to libraries and tools. |
Go
Go, also known as Golang, is an open-source programming language developed by Google. It was designed to provide a simpler approach to systems programming and enable efficient development of concurrent software. Go’s simplicity and built-in support for concurrency make it suitable for network programming and large-scale distributed systems.
Feature | Description |
---|---|
Concurrency | Go’s goroutines and channels simplify the development of concurrent software and communication between concurrent components. |
Performance | Go offers excellent runtime performance and efficient memory utilization. |
Community | With the backing of Google, Go has a growing community and comprehensive documentation. |
C#
C# (pronounced “C sharp”) is an object-oriented programming language developed by Microsoft. It is part of the .NET framework and is widely used for building Windows applications, web services, and game development with Unity. C# offers a modern syntax and powerful features, making it suitable for a variety of development scenarios.
Feature | Description |
---|---|
Productivity | C# emphasizes developer productivity with features like generics, LINQ, and asynchronous programming. |
Performance | C# applications have excellent performance, with the JIT compiler optimizing code at runtime. |
Community | C# benefits from the extensive resources and support provided by the .NET community and Microsoft. |
In conclusion, choosing the right programming language depends on various factors, including project requirements, personal preferences, and the availability of supporting libraries and frameworks. Each language presented here has its own unique characteristics and strengths, catering to different needs and application domains. It is crucial for developers to carefully evaluate and consider these factors before embarking on a new software development journey.
Frequently Asked Questions
What is input data in Python?
Input data in Python refers to the information or values provided to a program during runtime. It allows users to interact with the program by inputting data that can be processed, manipulated, or displayed.
How can I take user input in Python?
In Python, you can use the input()
function to take user input. This function prompts the user for input and returns the entered value as a string.
How do I convert user input to a specific data type?
To convert user input to a specific data type, you can use functions such as int()
, float()
, or bool()
. These functions can parse and convert the input string into the desired data type.
Can I take multiple inputs from a user in a single statement?
Yes, you can take multiple inputs from a user in a single statement by using the split()
method. By default, the split()
method splits the input string at whitespace and returns a list of strings.
How can I handle invalid input or errors?
You can handle invalid input or errors using exception handling in Python. By using try
, except
, and else
blocks, you can catch and handle specific errors raised by the program when dealing with user input.
Can I read input data from a file in Python?
Yes, Python provides functions to read input data from files. You can use the open()
function to open a file and then use methods like read()
or readlines()
to read the content of the file into your program.
How do I handle large input data sets efficiently?
To handle large input data sets efficiently, you can optimize your code by using appropriate data structures and algorithms. For example, using generators, reading files line by line instead of loading them entirely into memory, or implementing efficient data processing techniques.
Is there a way to validate user input?
Yes, you can validate user input by using regular expressions or by implementing custom functions to check if the input meets certain criteria. By defining validation rules, you can ensure the input data adheres to the expected format or constraints.
How can I securely handle sensitive user input, such as passwords?
For handling sensitive user input like passwords, it is recommended to use libraries or modules specifically designed for secure input handling. The getpass
module in Python provides a secure way to prompt users for passwords without displaying the input on the screen.
Can I store and retrieve user input for future use?
Yes, you can store and retrieve user input for future use by utilizing data storage options such as databases, files, or even variables within your program. By saving the input data, you can access and use it whenever required.