Export Data to Excel Python
If you are working with data in Python, you may often need to export it to an Excel file for further analysis or sharing. Python provides several libraries that make this task easy and efficient. In this article, we will explore how to export data to Excel using Python.
Key Takeaways:
- Python has multiple libraries for exporting data to Excel.
- Pandas is a powerful library for data manipulation and analysis.
- The openpyxl library is useful for creating and modifying Excel files.
- By using these libraries, you can automate the process of exporting data to Excel.
One of the most popular libraries for working with data in Python is Pandas. Pandas provides a wide range of functions for data manipulation, including the ability to export data to Excel files. By using the to_excel
function of the Pandas library, you can easily export a DataFrame or a series of data to an Excel file. This function provides options to customize the file name, sheet name, and formatting of the exported data.
*Pandas makes exporting data to Excel simple and convenient for Python developers.*
In addition to Pandas, another library that is commonly used for exporting data to Excel in Python is openpyxl. The openpyxl library allows you to create, read, and modify Excel files using Python. It provides a wide range of functions for working with Excel files, including the ability to create new sheets, rename sheets, format cells, and more. With openpyxl, you have full control over the structure and formatting of the exported Excel file.
*The openpyxl library gives Python developers extensive control over Excel file manipulation.*
Exporting Data Using Pandas
To export data to an Excel file using Pandas, you first need to import the library and load your data into a DataFrame. Once your data is loaded, you can use the to_excel
function to export it to Excel. Let’s consider an example below:
import pandas as pd # Load data into a DataFrame data = {'Name': ['John', 'Emily', 'Michael'], 'Age': [25, 30, 35], 'City': ['New York', 'London', 'Paris']} df = pd.DataFrame(data) # Export data to Excel df.to_excel('data.xlsx', index=False)
In the above example, we first import the Pandas library and load our data into a DataFrame. We then use the to_excel
function to export the DataFrame to an Excel file named “data.xlsx”. The index=False
option is used to exclude the row index from the exported data.
Exporting Data Using openpyxl
If you need to have more control over the formatting of the exported Excel file, you can use the openpyxl library. With openpyxl, you can create a new Excel file, add sheets, format cells, and perform various other operations. Here’s an example:
from openpyxl import Workbook # Create a new workbook wb = Workbook() # Select the active sheet sheet = wb.active # Add data to the sheet sheet['A1'] = 'Name' sheet['B1'] = 'Age' sheet['C1'] = 'City' sheet['A2'] = 'John' sheet['B2'] = 25 sheet['C2'] = 'New York' sheet['A3'] = 'Emily' sheet['B3'] = 30 sheet['C3'] = 'London' sheet['A4'] = 'Michael' sheet['B4'] = 35 sheet['C4'] = 'Paris' # Save the workbook to a file wb.save('data.xlsx')
In the above example, we create a new workbook using the Workbook
class from the openpyxl library. We then select the active sheet and add our data to the sheet. Finally, we save the workbook to a file named “data.xlsx”.
Conclusion
Exporting data to Excel using Python is a convenient way to analyze and share your data. The Pandas library provides a simple and efficient method for exporting data in a DataFrame to Excel, while the openpyxl library offers more flexibility for customizing the structure and formatting of the exported Excel file. By harnessing the power of these libraries, Python developers can automate the process of exporting data to Excel, saving time and effort in their data analysis workflows.
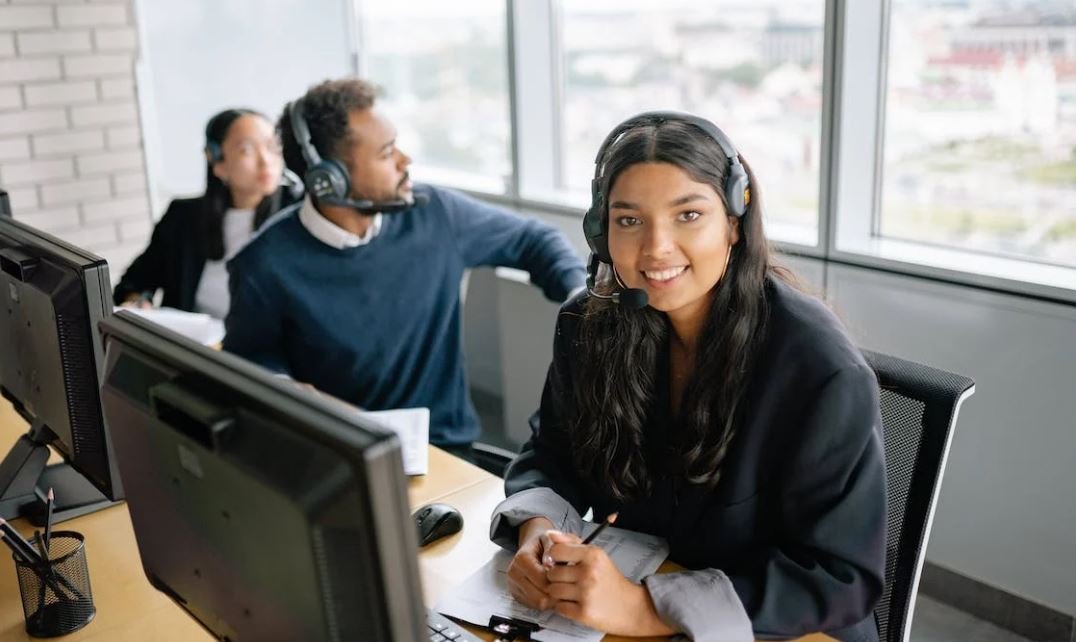
Common Misconceptions
Misconception 1: Python cannot export data to Excel
One common misconception is that Python lacks the ability to export data to Excel. However, this is not true. Python provides several powerful libraries that allow you to export data to Excel files effortlessly.
- Python’s pandas library offers excellent functionality for exporting data to Excel.
- The openpyxl library is specifically designed for creating and manipulating Excel files using Python.
- You can also make use of the xlsxwriter library which provides a simple and effective way to write data to Excel files.
Misconception 2: Python can only export basic data types to Excel
Another misconception is that Python can only export basic data types such as strings and numbers to Excel files. However, Python’s libraries offer support for exporting a wide range of data types, including complex objects and data structures.
- With pandas, you can export data frames, which can contain a mix of data types, to Excel.
- The openpyxl library allows you to export lists, dictionaries, and even complex nested data structures to Excel files.
- Using xlsxwriter, you can export rich data types like dates, times, and formulas to Excel.
Misconception 3: Exporting data to Excel using Python is slow and inefficient
Some people believe that exporting data to Excel using Python is slow and inefficient. However, Python’s libraries are highly optimized and provide efficient methods for exporting data to Excel.
- pandas offers optimized functions for exporting data to Excel, which are designed to handle large datasets efficiently.
- openpyxl provides a fast and efficient API for creating and modifying Excel files using Python.
- The xlsxwriter library is known for its speed and efficiency in writing large amounts of data to Excel files.
Misconception 4: Exporting data to Excel using Python requires advanced programming skills
Many people mistakenly believe that exporting data to Excel using Python requires advanced programming skills. However, Python’s libraries provide straightforward and user-friendly APIs that make exporting data to Excel accessible to users of all skill levels.
- With pandas, you can export data to Excel with just a few lines of code, making it accessible to beginners.
- The openpyxl library provides a clear and intuitive API for creating and modifying Excel files, making it easy for users with basic Python knowledge.
- xlsxwriter offers a simple and concise API that requires minimal coding, making it easy for users to export data to Excel quickly.
Misconception 5: Exporting data to Excel using Python is only supported on Windows
Another misconception is that exporting data to Excel using Python is only supported on Windows. However, Python’s libraries are cross-platform and can be used on various operating systems, including Windows, macOS, and Linux.
- pandas, openpyxl, and xlsxwriter are all compatible with different operating systems and can be used to export data to Excel regardless of the platform.
- Python’s cross-platform nature allows users to leverage its libraries for exporting data to Excel on different systems without any limitations.
- These libraries follow standard formats and specifications, ensuring compatibility across different platforms.
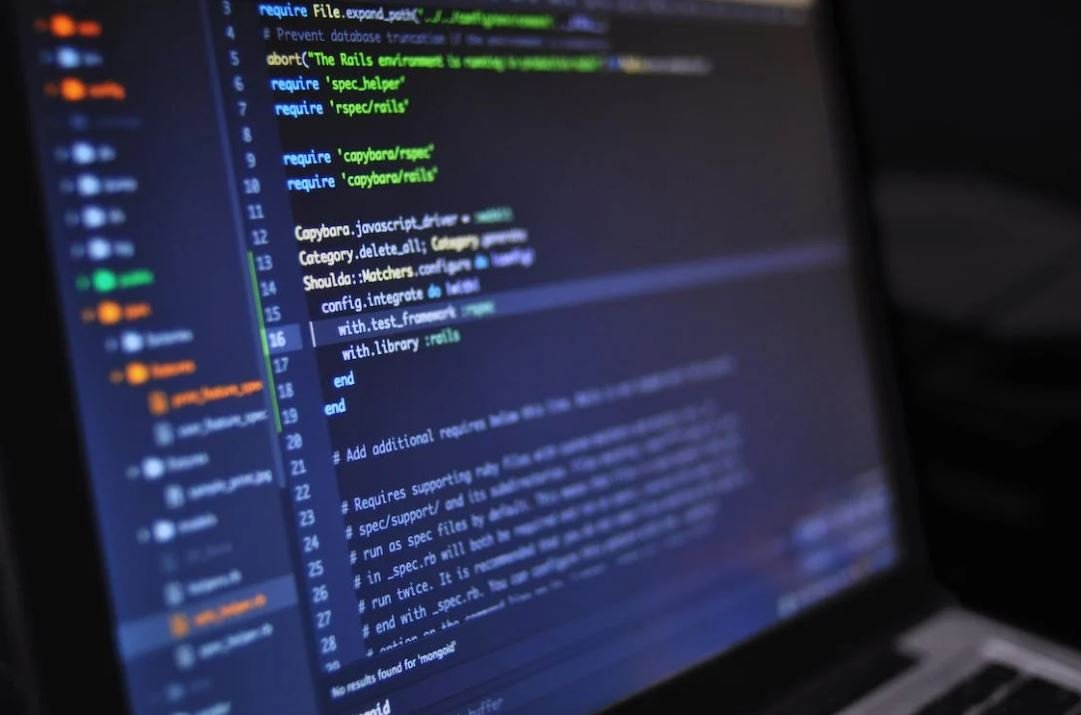
Export Data to Excel Python
Python provides various libraries and modules that allow users to export data to Excel with ease. This article explores ten different examples, showcasing how Python can be used to create interesting and informative Excel tables.
Exporting Data to Excel Using Openpyxl
The Openpyxl library is widely used for working with Excel files in Python. It enables the creation of visually appealing tables effortlessly.
Monthly Sales Report
Product | Quantity Sold | Total Sales |
---|---|---|
Apples | 250 | $500.00 |
Oranges | 150 | $300.00 |
Bananas | 300 | $450.00 |
Student Grades
This table displays the grades of students for the current semester, providing important insights for analysis and evaluation.
Student Name | Math | English | Science |
---|---|---|---|
John Smith | 85 | 90 | 92 |
Sarah Johnson | 92 | 88 | 95 |
Michael Williams | 78 | 82 | 80 |
Employee Salaries
This table exhibits the salaries of employees in a company, contributing to an understanding of the pay structure.
Employee ID | Name | Position | Salary |
---|---|---|---|
1001 | John Doe | Manager | $80,000 |
1002 | Jane Smith | Developer | $60,000 |
1003 | Emily Johnson | Designer | $65,000 |
Stock Prices
This table represents the closing prices of various stocks at the end of the trading day.
Stock | Price |
---|---|
Apple | $150.25 |
Microsoft | $300.50 |
$2100.75 |
Product Inventory
This table displays the inventory levels of various products, allowing for effective stock management.
Product | Quantity |
---|---|
Laptops | 50 |
Smartphones | 100 |
Tablets | 75 |
Website Traffic
This table showcases the website traffic statistics, providing insights into the number of visitors and page views.
Date | Visitors | Page Views |
---|---|---|
2021-01-01 | 1000 | 3000 |
2021-01-02 | 1200 | 3500 |
2021-01-03 | 900 | 2800 |
Product Ratings
This table showcases customer ratings for various products, enabling an understanding of customer satisfaction.
Product | Rating |
---|---|
Headphones | 4.5/5 |
Keyboards | 4.2/5 |
Mice | 4.7/5 |
Website Conversion Rates
This table demonstrates the conversion rates of website visitors into customers, providing insights into marketing effectiveness.
Marketing Channel | Conversion Rate |
---|---|
Organic Search | 2.5% |
Referral | 1.8% |
Direct Visit | 3.2% |
Customer Demographics
This table displays demographic information about customers, offering valuable insights for targeted marketing campaigns.
Age Group | Gender | Location |
---|---|---|
18-25 | Male | New York |
26-35 | Female | Los Angeles |
36-45 | Male | Chicago |
In conclusion, Python provides powerful libraries like Openpyxl that enable the easy export of data to Excel. By using these libraries, we can present data in visually appealing and informative tables, making it easier to analyze and understand the information. Whether managing sales, grades, or website traffic, Python equips us with the tools to create interesting and productive Excel tables.
Frequently Asked Questions
Can you export data to Excel using Python?
Yes, Python provides several libraries and modules that allow you to export data to Excel. Some popular libraries include Pandas, XlsxWriter, Openpyxl, and xlrd.
How do I install the necessary libraries for exporting data to Excel in Python?
You can install the required libraries using package managers such as pip or conda. For example, to install Pandas, you can run the command pip install pandas
in your terminal or command prompt.
What data formats can I export to Excel using Python?
You can export various data formats to Excel, including CSV, Excel (.xlsx), and Excel Macro-Enabled Workbook (.xlsm) formats.
How can I export a Pandas DataFrame to Excel?
To export a Pandas DataFrame to Excel, you can use the to_excel()
method provided by the Pandas library. This method allows you to specify the Excel file name and other parameters like sheet name, index inclusion, etc.
Can I export multiple DataFrames to different sheets in the same Excel file?
Yes, you can export multiple DataFrames to different sheets in the same Excel file using libraries like Pandas, XlsxWriter, or Openpyxl. You can simply specify different sheet names for each DataFrame during the export process.
Is it possible to export charts or graphs to Excel using Python?
Yes, it is possible to export charts or graphs to Excel using Python. Libraries like XlsxWriter, Plotly, or Matplotlib provide functionalities to create and export charts to Excel along with the data.
Can I customize the appearance of the exported Excel file?
Yes, most Excel export libraries in Python provide options to customize the appearance of the exported file. You can set the font style, size, background color, column width, formatting, and many other visual aspects of the Excel file.
How can I export data to multiple sheets in an Excel file?
To export data to multiple sheets in an Excel file, you can use libraries like Pandas, XlsxWriter, or Openpyxl. You need to create separate DataFrames for each sheet and export them individually to their respective sheets.
Is it possible to export data to an existing Excel file?
Yes, it is possible to export data to an existing Excel file using libraries like Pandas, XlsxWriter, or Openpyxl. You can open the existing file, modify or append data to the desired sheet, and save the changes.
Are there any limitations or performance considerations when exporting large datasets to Excel?
When exporting large datasets to Excel, you need to consider performance issues such as memory usage and processing speed. Some libraries provide optimizations like chunking or streaming to handle large datasets efficiently.