Data Input and Output in C
In the C programming language, data input and output (I/O) operations play a crucial role in handling user interactions and managing files. Understanding how to effectively perform I/O tasks in C is essential for developers looking to build robust and interactive programs. This article will explore the various techniques and functions available in C for data input and output, providing you with the knowledge to handle I/O operations with ease.
Key Takeaways
- Understanding data input and output in C is essential for handling user interactions and file management.
- C provides several I/O functions, including those for standard input and output, file I/O, and formatted I/O.
- Error handling is crucial when performing I/O operations in C to ensure the integrity of the program.
- Using buffering techniques can significantly improve the efficiency of data I/O in C programs.
Standard Input and Output
The C programming language provides three standard I/O streams: stdin, stdout, and stderr. These streams are automatically opened by the C runtime environment and are accessible by default. They allow for input from the user (stdin) and output to the console (stdout) and error messages (stderr).
Using the standard I/O streams in C enables developers to interact with users and display feedback in the console.
File I/O
In addition to standard I/O, C also provides functions for performing I/O operations on files. The stdio.h header file contains essential functions like fopen(), fclose(), fread(), and fwrite() that allow you to open, close, read, and write data to files, respectively.
File I/O in C enables developers to read data from files, write data to files, and manipulate files as needed.
Formatted I/O
C provides sprintf() and sscanf() functions for performing formatted I/O. These functions allow you to read and write data in a specific format, such as integers or floating-point numbers.
Formatted I/O functions in C are useful for parsing input or outputting data in a particular format.
Buffering
Buffering data during I/O operations can significantly improve the performance of C programs. C uses buffering techniques, such as input buffering and output buffering, to minimize the number of actual I/O operations.
Buffering data in C helps reduce the overhead of frequent I/O requests and enhances program execution speed.
Table 1: I/O Functions in C
Function | Description |
---|---|
printf() | Prints formatted data to the console (stdout). |
scanf() | Reads data from standard input (stdin) based on the specified format. |
fopen() | Opens a file in the specified mode for file I/O operations. |
fclose() | Closes the specified file after performing file I/O operations. |
fgets() | Reads a line from a file and stores it as a string. |
Table 2: File I/O Modes
Mode | Description |
---|---|
r | Opens a file for reading. |
w | Opens a file for writing (creates a new file if not found, truncates existing file). |
a | Opens a file for appending data at the end (creates a new file if not found). |
Table 3: Formatting Specifiers
Specifier | Description |
---|---|
%d | Represents an integer value. |
%f | Represents a floating-point value. |
%c | Represents a character. |
%s | Represents a string. |
By mastering data input and output in C, developers can create programs that efficiently handle user interactions and manage files effectively. Whether it’s displaying output to the console, reading from files, or working with formatted data, understanding the various techniques and functions available will empower you to build powerful C programs.
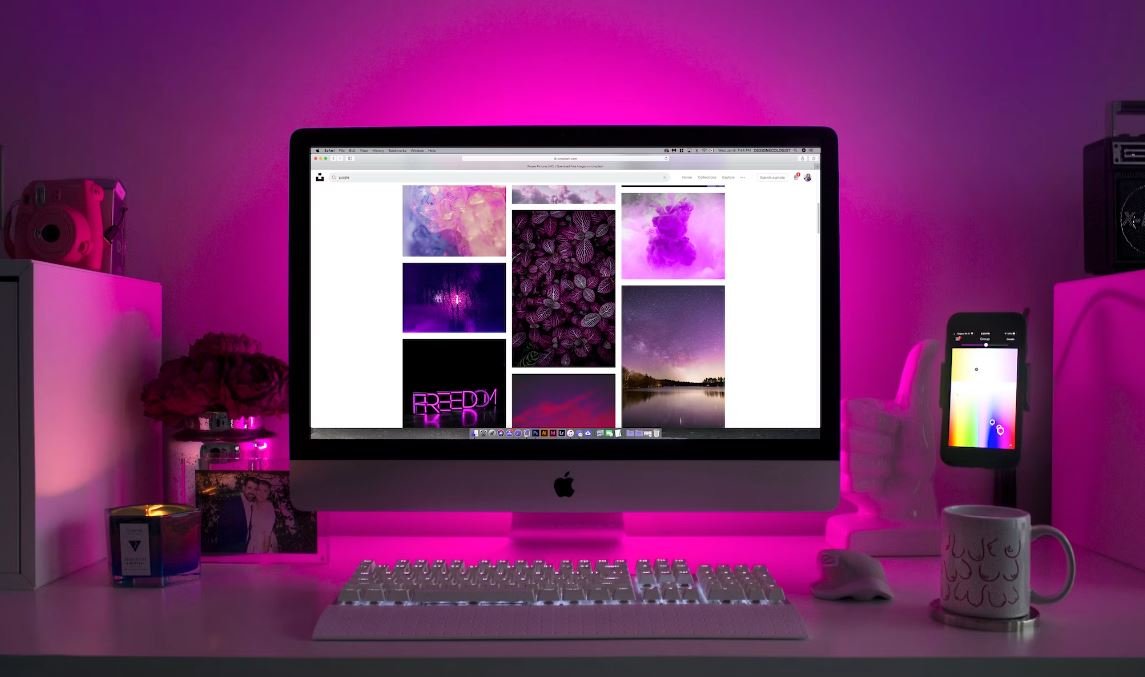
Common Misconceptions
Data Input and Output in C
There are a few common misconceptions surrounding data input and output in the C programming language. Let’s debunk them:
- Input and output in C is only limited to the console: While the console is commonly used for input and output in C, it is not the only option. C provides various functions and libraries that allow for input and output from files, network sockets, and other devices.
- Data input in C can be performed by the user at any time: Unlike some other programming languages, C is a sequential language. To input data, the program needs to explicitly request input from the user using specific input functions or methods.
- Data output in C is always displayed immediately: In C, the output can be stored in variables or buffers before being displayed on the screen or saved into a file. This allows for formatting and processing of the data before it is presented to the user.
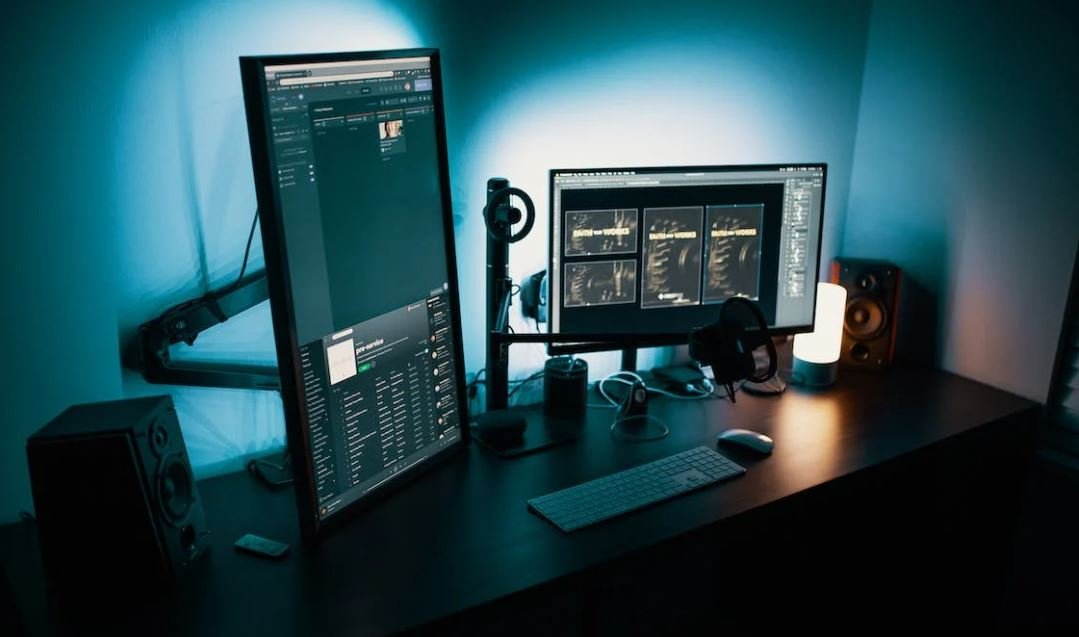
Data Input and Output in C
In the field of programming, data input and output (I/O) are crucial aspects to consider when designing applications. In the C programming language, various techniques are employed to perform efficient and reliable data input and output operations. This article explores different approaches for data input and output in C and showcases their practical applications. Each table below provides an in-depth analysis and examples of these techniques, highlighting their strengths and demonstrating their use in real-world scenarios.
Using printf() for Output
The printf() function in C is a versatile tool for displaying information to the user. By using different format specifiers, you can output various data types, such as integers, characters, and strings. The table below showcases the usage of printf() for different data types.
Data Type | Format Specifier | Output Example |
---|---|---|
Integer | %d | 42 |
Character | %c | A |
String | %s | Hello, World! |
Using scanf() for Input
The scanf() function in C is commonly used to read input from the user. It allows you to assign values to variables based on the user’s input. The table below demonstrates the usage of scanf() to obtain different types of input.
Data Type | Format Specifier | User Input Example |
---|---|---|
Integer | %d | 56 |
Character | %c | X |
String | %s | Input received |
File I/O Operations
Working with files is a fundamental part of many applications. In C, file I/O operations allow you to read from and write to files. The table below presents different file I/O functions and their applications.
Function | Description | Example |
---|---|---|
fopen() | Opens a file for subsequent operations | fopen(“data.txt”, “r”) |
fread() | Reads data from a file | fread(buffer, sizeof(char), 100, file) |
fwrite() | Writes data to a file | fwrite(data, sizeof(char), 10, file) |
Error Handling for I/O Operations
When performing data input and output, error handling is essential to ensure that operations are successful and to handle any potential issues. The table below showcases common error codes and their corresponding meanings in C.
Error Code | Meaning |
---|---|
0 | Operation succeeded |
1 | Operation failed |
-1 | End of file reached |
Binary I/O vs. Text I/O
When working with files, you have the option to use binary I/O or text I/O. The table below outlines the differences between these two approaches.
Aspect | Binary I/O | Text I/O |
---|---|---|
Representation | Raw binary data | Human-readable text |
Size | Smaller | Larger |
Portability | Less portable | More portable |
Sequential and Random File Access
Depending on the application requirements, you may need to perform sequential or random access to files. The table below illustrates the differences between these two approaches.
Aspect | Sequential Access | Random Access |
---|---|---|
Read/Write Order | Sequentially from start to end | Any order |
Speed | Faster for large files | Faster for small data retrieval |
Flexibility | Less flexible | More flexible |
Console I/O vs. File I/O
In C, you can perform input and output operations through the console (standard input/output) or files. The table below compares the characteristics of console I/O and file I/O.
Aspect | Console I/O | File I/O |
---|---|---|
Interaction | User input/output | Read/write files |
Availability | Always available | Depends on file existence |
Persistence | Transient | Persistent |
Formatted vs. Unformatted I/O
In C, you have the option to perform formatted or unformatted input/output operations. The table below highlights the differences between these two approaches.
Aspect | Formatted I/O | Unformatted I/O |
---|---|---|
Human Readability | Readable text | Not easily readable |
Output Precision | Controlled formatting | Binary representation |
Data Parsing | Easier parsing | Custom parsing required |
In conclusion, mastering data input and output techniques in C is essential for designing robust and efficient applications. Understanding printf() and scanf() usage, file I/O operations, error handling, and the various choices available for input/output methods allows developers to effectively handle data in their programs. Whether it’s through console or file, formatted or unformatted, understanding the characteristics of each approach enables programmers to make informed decisions and build powerful software.
Frequently Asked Questions
How do I prompt the user for input in C?
Your program can prompt the user for input using the printf
function to display a message to the user, followed by the scanf
function to read in the input from the user.
What is the syntax for using the scanf
function in C?
The syntax for using the scanf
function in C is: scanf(format, &variable)
, where format
specifies the type of data to be read and &variable
is the memory address of the variable to store the input.
How do I read input from a file in C?
To read input from a file in C, you can use the fscanf
function. This function works similarly to scanf
, but you need to specify the file pointer as the first argument.
How do I write output to the console in C?
You can use the printf
function to write output to the console in C. Simply pass the desired output as a string argument to printf
.
How do I write output to a file in C?
To write output to a file in C, you can use the fprintf
function. This function works similar to printf
, but you need to specify the file pointer as the first argument.
What is the difference between fprintf
and printf
in C?
The fprintf
function is used to write output to a file, while printf
is used to write output to the console. The syntax and functionality of both functions are the same, except that fprintf
requires an additional argument specifying the file pointer.
How do I read and write binary files in C?
To read and write binary files in C, you can use the fread
and fwrite
functions respectively. These functions allow you to read or write binary data directly without any formatting.
What is file buffering in C?
File buffering in C is a mechanism that temporarily stores the data read from or to be written to a file. It helps optimize input/output operations by reducing the number of physical reads or writes performed on the file. C provides functions like setbuf
and setvbuf
to control the buffering mode.
How do I handle errors while performing input or output operations in C?
You should always check the return values of input/output functions like fscanf
, fprintf
, and others to detect any errors. When an error occurs, these functions usually return a value that indicates the error. You can use error handling techniques like conditional statements or error codes to handle errors appropriately.
What is file truncation in C?
File truncation in C is the process of shrinking the size of a file by removing a portion of its data. This can be done using the ftruncate
function, which allows you to specify the new size of the file. Truncating a file can be useful when you need to update or delete some data from the file.