Computer Science Algorithms Exam Questions
Are you studying computer science and seeking practice questions to solidify your understanding of algorithms? This article provides a comprehensive collection of exam questions that cover various topics in computer science algorithms.
Key Takeaways:
- Prepare for your computer science algorithms exams with these practice questions.
- Deepen your understanding of fundamental algorithms and their implementation.
- Gain confidence by solving different types of algorithmic problems.
Question 1:
In the Merge Sort algorithm, explain the following steps:
- Divide the unsorted list into sublists.
- Repeatedly merge the sublists together.
- Continue merging until there is only one sorted list.
Question 2:
What are the time and space complexities of the QuickSort algorithm?
The time complexity is O(n log n) in average and best cases, while the worst case time complexity is O(n^2). The space complexity is O(log n).
Table 1: Sorting Algorithms Comparison
Algorithm | Time Complexity | Stability |
---|---|---|
Bubble Sort | O(n^2) | Stable |
Selection Sort | O(n^2) | Unstable |
Insertion Sort | O(n^2) | Stable |
Question 3:
What is the purpose of Dijkstra’s algorithm and how does it work?
Dijkstra’s algorithm is used to find the shortest path between two vertices (or nodes) in a graph. It works by iteratively selecting the vertex with the smallest distance from the source vertex and updating the distances of its neighbors.
Question 4:
Compare and contrast Breadth-First Search (BFS) and Depth-First Search (DFS).
- BFS explores nodes level by level, while DFS explores nodes branch by branch.
- BFS uses a queue data structure, while DFS uses a stack or recursion.
- BFS guarantees the shortest path, while DFS may not find the shortest path.
Table 2: Sorting Algorithms Time Complexity
Algorithm | Best Case | Average Case | Worst Case |
---|---|---|---|
Merge Sort | O(n log n) | O(n log n) | O(n log n) |
QuickSort | O(n log n) | O(n log n) | O(n^2) |
Heap Sort | O(n log n) | O(n log n) | O(n log n) |
Question 5:
What is the purpose of the Knapsack problem and how does it relate to dynamic programming?
The Knapsack problem involves optimizing the selection of items to include in a knapsack given their weights and values. It is commonly solved using dynamic programming techniques, such as memoization or tabulation.
Question 6:
How does the Floyd-Warshall algorithm work and what is its time complexity?
The Floyd-Warshall algorithm is used to find the shortest paths between all pairs of vertices in a weighted graph. It works by constructing a distance matrix and updating it iteratively. The time complexity of the algorithm is O(V^3), where V is the number of vertices.
Table 3: Sorting Algorithms Stability
Algorithm | Stable |
---|---|
Merge Sort | Yes |
QuickSort | No |
Heap Sort | No |
With these practice questions, you can strengthen your knowledge and enhance your problem-solving skills in computer science algorithms. Remember to thoroughly understand the concepts and analyze the pros and cons of different algorithms. Happy studying!
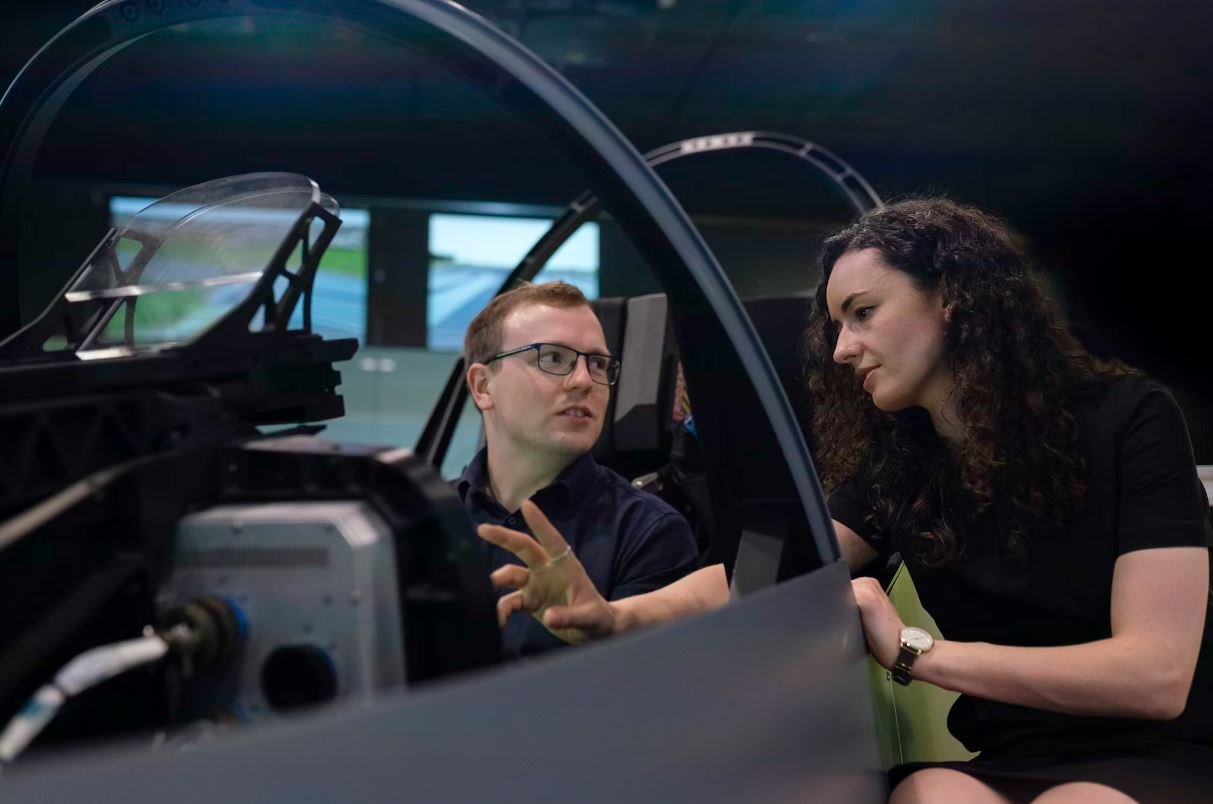
Common Misconceptions
Misconception 1: Computer Science Algorithms Exam Questions are Only for Geniuses
Contrary to popular belief, computer science algorithms exam questions are not only for geniuses. While these exams may require logical thinking and problem-solving skills, anyone can excel with the right amount of preparation and practice. It is important to understand that success in this field is not solely determined by innate intelligence.
- Preparation and practice are key to success in computer science algorithms exams.
- Logical thinking and problem-solving skills can be developed and improved with time and effort.
- Success in computer science algorithms exams is not exclusively reserved for geniuses.
Misconception 2: Memorization is Sufficient for Computer Science Algorithms Exams
Many people mistakenly believe that memorization of algorithms is enough to succeed in computer science algorithms exams. While having a good understanding of various algorithms is important, it is equally crucial to be able to apply them to solve different problems. These exams typically test the ability to analyze, design, and implement algorithms, rather than regurgitate memorized information.
- Understanding and application of algorithms are essential for success in computer science algorithms exams.
- Memorization alone is not sufficient; the ability to solve problems using algorithms is crucial.
- Exams focus on analyzing, designing, and implementing algorithms, not simple regurgitation.
Misconception 3: Computer Science Algorithms Exams Rely Heavily on Coding
One misconception about computer science algorithms exams is that they heavily rely on coding. While coding is certainly a part of these exams, they also assess other important aspects, such as algorithm analysis, complexity analysis, and problem decomposition. It is important to have a well-rounded understanding of different aspects of algorithms, rather than just focusing on coding skills.
- Computer science algorithms exams assess various aspects, not just coding skills.
- Algorithm analysis, complexity analysis, and problem decomposition are equally important in these exams.
- A well-rounded understanding of different aspects of algorithms is crucial for success.
Misconception 4: Theoretical Knowledge is Enough for Computer Science Algorithms Exams
Another common misconception is that theoretical knowledge alone is sufficient to excel in computer science algorithms exams. While having a strong theoretical foundation is important, practical problem-solving skills are equally crucial. These exams often require applying theoretical knowledge to real-world scenarios and developing efficient algorithms to solve complex problems.
- Practical problem-solving skills are just as important as theoretical knowledge in computer science algorithms exams.
- The ability to apply theoretical knowledge to real-world scenarios is a key aspect of the exams.
- Developing efficient algorithms to solve complex problems is a crucial skill to possess.
Misconception 5: Computer Science Algorithms Exams Only Test Knowledge, not Skills
Some people mistakenly believe that computer science algorithms exams are purely knowledge-based and do not assess practical skills. However, these exams are designed to assess both knowledge and skills. While understanding the concepts is important, the ability to apply that knowledge to solve problems is equally vital. These exams often require critical thinking and creative problem-solving skills.
- Computer science algorithms exams assess both knowledge and practical skills.
- Problem-solving abilities, critical thinking, and creativity are important in these exams.
- Understanding the concepts is crucial, but the application of that knowledge is equally vital.
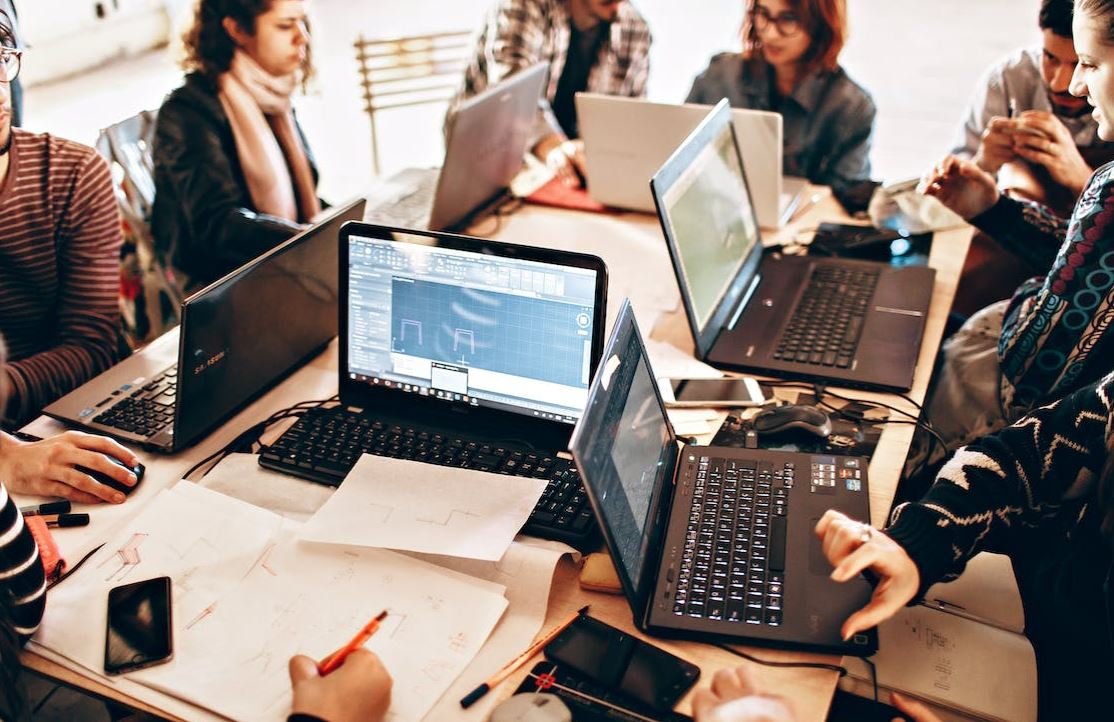
Table: Most Popular Sorting Algorithms
In computer science, sorting algorithms are used to arrange data in a particular order. This table highlights some of the most commonly used sorting algorithms along with their average time complexity.
Algorithm | Average Time Complexity |
---|---|
Bubble Sort | O(n^2) |
Selection Sort | O(n^2) |
Insertion Sort | O(n^2) |
Merge Sort | O(n log n) |
Quick Sort | O(n log n) |
Heap Sort | O(n log n) |
Radix Sort | O(nk) |
Table: Comparative Analysis of Graph Traversal Algorithms
Graph traversal algorithms are used to explore or search through a graph data structure. This table presents a comparison of some popular graph traversal algorithms based on their characteristics.
Algorithm | Time Complexity | Space Complexity | Advantages | Disadvantages |
---|---|---|---|---|
Breadth-First Search (BFS) | O(V + E) | O(V) | Guaranteed to find the shortest path in an unweighted graph | Requires more memory due to the use of a queue |
Depth-First Search (DFS) | O(V + E) | O(V) | Memory-efficient as it uses a stack | May get trapped in infinite loops in infinite graphs |
Dijkstra’s Algorithm | O((V + E) log V) | O(V) | Finds the shortest path in weighted graphs | Does not work with negative edge weights |
Table: Run-time Analysis of Dynamic Programming Algorithms
Dynamic programming is a method for solving complex problems by breaking them down into simpler overlapping subproblems. This table presents the run-time complexity of various dynamic programming algorithms.
Algorithm | Time Complexity |
---|---|
Fibonacci | O(n) |
Knapsack | O(nW) |
Longest Common Subsequence | O(mn) |
Table: Comparison of Search Algorithms
Search algorithms are used to find a specific element or item within a given collection of data. This table compares the time complexity and characteristics of different search algorithms.
Algorithm | Time Complexity | Advantages | Disadvantages |
---|---|---|---|
Linear Search | O(n) | Simple, applicable for both sorted and unsorted data | Inefficient for large datasets |
Binary Search | O(log n) | Faster than linear search for sorted data | Requires the data to be sorted |
Hashing | O(1) | Constant-time access | May lead to collisions |
Table: Space Complexity of Popular Data Structures
Data structures are used to organize and store data efficiently. This table showcases the space complexity of some commonly used data structures.
Data Structure | Space Complexity |
---|---|
Array | O(n) |
Linked List | O(n) |
Stack | O(n) |
Queue | O(n) |
Binary Search Tree | O(n) |
Heap | O(n) |
Hash Table | O(n) |
Table: Average Case Time Complexity for Matrix Multiplication Algorithms
Matrix multiplication is an essential operation in various scientific and engineering applications. This table presents the average case time complexity of different matrix multiplication algorithms.
Algorithm | Average Time Complexity |
---|---|
Naive Algorithm | O(n^3) |
Strassen’s Algorithm | O(n^log2(7)) ≈ O(n^2.81) |
Coppersmith-Winograd Algorithm | O(n^2.376) |
Table: Analysis of Divide and Conquer Algorithms
Divide and conquer is a technique to solve problems by dividing them into smaller subproblems, solving the subproblems recursively, and then combining the results. This table provides insight into the time and space complexities of various divide and conquer algorithms.
Algorithm | Time Complexity | Space Complexity |
---|---|---|
Merge Sort | O(n log n) | O(n) |
Quick Sort | O(n log n) | O(log n) |
Karatsuba Multiplication | O(n^log2(3)) ≈ O(n^1.585) | O(log n) |
Table: Analysis of Greedy Algorithms
Greedy algorithms make locally optimal choices to reach a global optimum in problem-solving. This table examines the time complexity and characteristics of several popular greedy algorithms.
Algorithm | Time Complexity | Advantages | Disadvantages |
---|---|---|---|
Activity Selection | O(n log n) | Easier to implement, provides an optimal solution for certain problems | May not always provide the globally optimal solution |
Huffman Coding | O(n log n) | Produces an optimal prefix-free code for encoding data | Requires knowledge of probability distribution |
Table: Comparison of Various Tree Traversal Algorithms
Tree traversal algorithms are used to visit all nodes in a tree data structure. This table compares the time complexity and characteristics of different tree traversal algorithms.
Algorithm | Time Complexity | Types | Advantages | Disadvantages |
---|---|---|---|---|
Pre-order | O(n) | Pre, In, Post | Can be used to create a copy of the tree | Does not provide the exact structure of the tree |
In-order | O(n) | Pre, In, Post | Provides nodes in ascending order for binary search trees | Does not preserve the root position |
Post-order | O(n) | Pre, In, Post | Can be used to delete the tree | Does not provide the exact structure of the tree |
Computer science algorithms play a vital role in solving complex problems efficiently and optimizing the use of computing resources. This article explored various aspects of algorithm design, including sorting, searching, dynamic programming, graph traversal, and more. Each table provided valuable insights into the different algorithms’ time and space complexities, advantages, and limitations. By understanding and implementing these algorithms, computer scientists can develop efficient solutions across a wide range of domains, driving advancements in technology and problem-solving capabilities.
Frequently Asked Questions
Computer Science Algorithms Exam Questions
FAQs
What are computer science algorithms?
Computer science algorithms refer to step-by-step procedures or instructions designed to solve specific problems or perform certain tasks in the field of computer science. These algorithms are used to manipulate data, process information, and perform various computations.
Why are algorithms important in computer science exams?
Algorithms form the foundation of computer science and are crucial for understanding and implementing efficient solutions to complex problems. They are extensively tested in computer science exams to assess students’ knowledge and problem-solving abilities.
What should I expect in a computer science algorithms exam?
A computer science algorithms exam typically consists of theoretical questions related to algorithm design principles, analysis, complexity, and data structures. It may also involve practical programming problems where you need to implement or analyze algorithms.
How can I prepare for a computer science algorithms exam?
To prepare for a computer science algorithms exam, it is essential to understand the core concepts and principles of algorithm design, analysis, and data structures. Practice solving algorithmic problems, implement algorithms in programming languages, and review relevant theoretical material.
What are some common types of algorithms tested in computer science exams?
Common types of algorithms tested in computer science exams include sorting algorithms (e.g., bubble sort, merge sort), searching algorithms (e.g., binary search, linear search), graph algorithms (e.g., depth-first search, breadth-first search), and dynamic programming algorithms.
Can you provide examples of algorithm exam questions?
While specific examples may vary, some common algorithm exam questions may involve analyzing the time complexity of an algorithm, designing an algorithm to solve a specific problem, applying an algorithm to manipulate data or perform operations, or identifying the correct output of a given algorithm.
How should I approach algorithm exam questions?
When approaching algorithm exam questions, read the instructions carefully, analyze the problem or task, and determine the appropriate algorithmic approach. Consider the time and space complexity, perform necessary calculations or steps, and clearly present the solution using appropriate pseudocode or programming code.
How can I improve my algorithmic problem-solving skills?
Improving algorithmic problem-solving skills requires consistent practice. Solve a variety of algorithmic problems, participate in coding challenges or competitions, study different algorithmic techniques, and analyze existing algorithms and their implementations to gain insights.
Are there any recommended resources for studying algorithms?
Yes, there are several recommended resources for studying algorithms, including textbooks such as ‘Introduction to Algorithms’ by Cormen, Leiserson, Rivest, and Stein, online algorithm courses on platforms like Coursera or edX, and algorithm visualization tools that help understand algorithmic processes visually.
What if I struggle with understanding or solving algorithmic problems?
If you struggle with understanding or solving algorithmic problems, seek additional resources or assistance. Consult your professor, join study groups or forums where you can discuss problems and solutions with peers, and utilize online platforms with explanations and practice problems specifically focused on algorithms.